Hello my friends, welcome back to my blog. Today in this blog post, I am going to show you, Laravel 9 Vue 3 Crud Tutorial Working Example – Add Edit Delete.
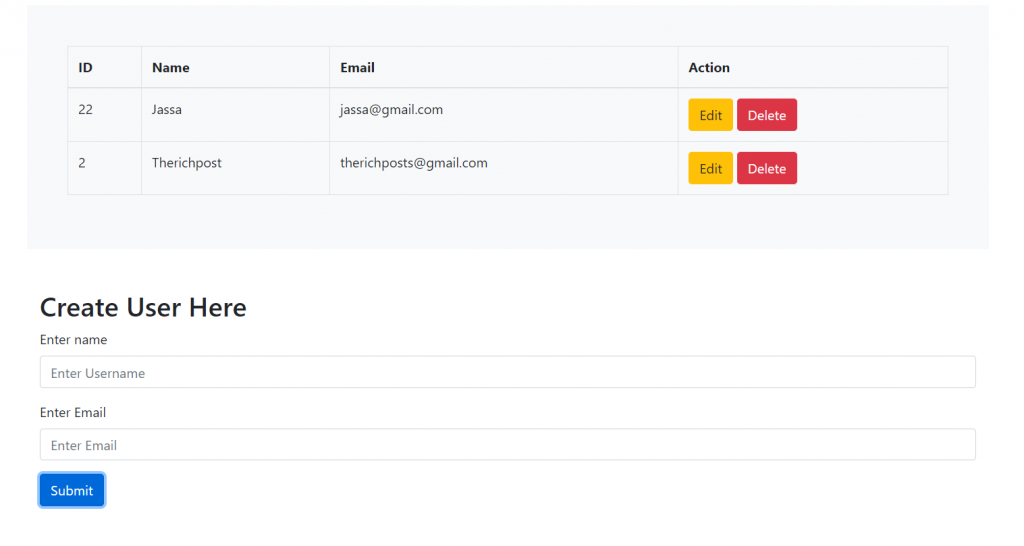
Vuejs3 came and if you are new then you must check below link::
Vuejs
Friends now I proceed onwards and here is the code snippet and please use this carefully to avoid the mistakes:
1. Firstly friends we need fresh vuejs(Vue 3) setup and for that we need to run below commands into our terminal and also w should have latest node version installed on our system:
Guys you can skip this first step if you already have vuejs fresh setup:
npm install -g @vue/cli vue create vuecrud cd vuecrud npm install bootstrap --save npm install axios npm run serve //http://localhost:8080/
2. Finally guys we need to add below code into our src/App.vue file to get final output on web browser:
<template> <div class="container mb-5 mt-5"> <div class="row"> <div class="col-md-12 bg-light p-5 mb-5"> <table class="table table-bordered"> <thead> <tr> <th scope="col">ID</th> <th scope="col">Name</th> <th scope="col">Email</th> <th scope="col">Action</th> </tr> </thead> <tbody> <tr v-for="post in posts" :key="post.id"> <td scope="row">{{post.id}}</td> <td>{{post.name}}</td> <td>{{post.email}}</td> <td><a href="#" class="btn btn-warning" @click="get_user(post.id,post.name,post.email)">Edit</a> <a href="#" class="btn btn-danger" @click="delete_user(post.id)">Delete</a></td> </tr> </tbody> </table> </div> <div class="col-md-12" v-if="adduser"> <h2>Create User Here</h2> <form v-on:submit.prevent="create_user"> <div class="form-group"> <label for="name">Enter name</label> <input type="text" name=" name" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter Username" v-model="form.name"> </div> <div class="form-group"> <label for="email">Enter Email</label> <input type="email" name=" email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter Email" v-model="form.email"> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> </div> <div class="col-md-12" v-if="edituser"> <h2>Edit User</h2> <form v-on:submit.prevent="update(upd_user.id)"> <div class="form-group"> <label for="name">Enter name</label> <input type="text" name=" name" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter Username" v-model="upd_user.name"> </div> <div class="form-group"> <label for="email">Enter Email</label> <input type="email" name=" email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp" placeholder="Enter Email" v-model="upd_user.email"> <input type="hidden" name="id" v-model="upd_user.id"> </div> <button type="submit" class="btn btn-primary">Update</button> </form> </div> </div> </div> <div class="jumbotron text-center mb-0"> <p>Footer</p> </div> </template> <script> import 'bootstrap/dist/css/bootstrap.min.css'; import axios from 'axios' export default { mounted () { //getting user data this.loadlist(); }, data(){ return { posts:[], edituser:false, adduser:true, form:{ name: '', email: '' }, upd_user:{ id:null, name:'', email:'', }, } }, methods:{ //getting all users details loadlist(){ axios .get('http://127.0.0.1:8000/users') .then((resp) => { console.log(resp); this.posts = resp.data; }) }, //add new user create_user(){ axios .post('http://127.0.0.1:8000/create',this.form) .then((resp) =>{ console.log(resp); this.loadlist(); //reset form this.form.name = ''; this.form.email = ''; }) .catch((e)=>{ console.log(e); }) }, //get user dtails to show inside edit form get_user(id,name,email){ this.edituser = true, this.adduser = false console.log(id,name,email); this.upd_user.id = id; this.upd_user.name = name; this.upd_user.email = email; }, //update user update(id){ console.log(id); axios .post('http://127.0.0.1:8000/user/update/'+id,this.upd_user ) .then((resp) =>{ console.log(resp); this.edituser = false, this.adduser = true this.loadlist(); }) .catch((e)=>{ console.log(e); }) }, //delete user delete_user(deleteid){ axios.delete('http://127.0.0.1:8000/user/delete/'+deleteid) .then((res) =>{ console.log(res); this.loadlist(); }) .catch((e)=>{ console.log(e); }) } }, } </script>
Guys here is Laravel 9 code snippet:
1. Guys, here is the code for Crud API’s(add, edit, view and delete) and we need to add into our Laravel 9 project routes/api.php file:
<?php ... Route::get('users','APIApiTestingController@index'); Route::match(['get','post'],'create/','APIApiTestingController@create'); Route::match(['get','post'],'user/{id}','APIApiTestingController@edit'); Route::match(['put','post'],'user/update/{id}','APIApiTestingController@update'); Route::delete('user/delete/{id}','APIApiTestingController@destroy'); ...
2. Guys we need to make new folder name “API” inside app/Http/Controllers/ folder and after it we need to make new file inside that “API” folder name ApiTestingController.php and add below code inside it:
<?php namespace AppHttpControllersAPI; use AppHttpControllersController; use IlluminateHttpRequest; use AppUser; class ApiTestingController extends Controller { public function index(){ //get all the users details $users = User::orderBy('id','desc')->get(); return $users; } public function create(Request $request){ //add new user if ($request->isMethod('post')) { $user = User::create(array( 'name' => $request['name'], 'email' => $request['email'], )); return $user; } } public function edit($id){ //get single user details $user = User::findOrfail($id); return $user; } public function update(Request $request, $id) { //update user data if ($request->isMethod('post')) { $user = User::findOrfail($id); $user->name = $request->get('name'); $user->email = $request->get('email'); $user->save(); return $user; } } public function destroy($id){ //delete user $user = User::findOrfail($id); $user->delete(); return response()->json([ 'message' => 'delete success', ]); } }
Now we are done friends also and If you have any kind of query or suggestion or any requirement then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. I will come with more demo which will helpful to all.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks
The post Laravel 9 Vue 3 Crud Tutorial Working Example – Add Edit Delete appeared first on Therichpost.
This content was originally published here.