In 2025, JavaScript Alerts in Capybara remain quite relevant, especially for testing web applications that use JavaScript extensively. According to StackShare, 96 companies use Capybara in their tech stacks, including well-known names like Hivebrite, Livestorm, and Festalab.
While modern web development has moved towards more sophisticated UI elements like modals and custom pop-ups, traditional JavaScript alerts, confirms, and prompts are still used in many applications.
Capybara provides robust methods to handle these alerts, making it easier to write tests that simulate user interactions with these pop-ups. This is particularly useful for ensuring that your application behaves correctly when these alerts are triggered.
Table of Contents
1/ Introduction on JavaScript Alerts in Capybara
Even with evolving web development trends, testing core functionalities remains crucial. In 2025, JavaScript’s alert()
, confirm()
, and prompt()
functions, despite the prevalence of more visually sophisticated UI elements, still provide essential user interaction and feedback mechanisms.
Often used alongside Ruby on Rails because it is a popular web-based test automation tool written in Ruby, Capybara, a robust acceptance testing framework, supports testing these JavaScript dialogs, offering methods like accept_alert
, dismiss_confirm
, and accept_prompt
to simulate user interactions. This ensures correct application behavior and a seamless user experience when alerts are triggered.
Testing these interactions is vital for:
- User Experience: Alerts often convey critical information or require user decisions, impacting user experience.
- Error Handling: Alerts can display error messages or warnings, requiring proper testing.
- Application Flow: Alerts can interrupt user actions, so testing their impact on application behavior and recovery is essential.
While modern UI elements offer enhanced visuals, JavaScript alerts remain significant for conveying information and prompting user decisions. Capybara’s continued support ensures developers can maintain web application reliability and functionality.
2/ Understanding JavaScript Dialogs
JavaScript provides three built-in dialog functions: alert()
, confirm()
, and prompt()
.
alert()
displays a message with an “OK” button, suitable for simple notifications.confirm()
presents a message with “OK” and “Cancel” buttons, ideal for actions requiring confirmation. It returnstrue
for “OK” andfalse
for “Cancel.”prompt()
displays a message with a text input field, “OK,” and “Cancel” buttons, used to gather user input. It returns the entered text on “OK” andnull
on “Cancel.”
While useful, excessive use of these functions can be disruptive. Consider alternatives like inline messages for a smoother user experience.
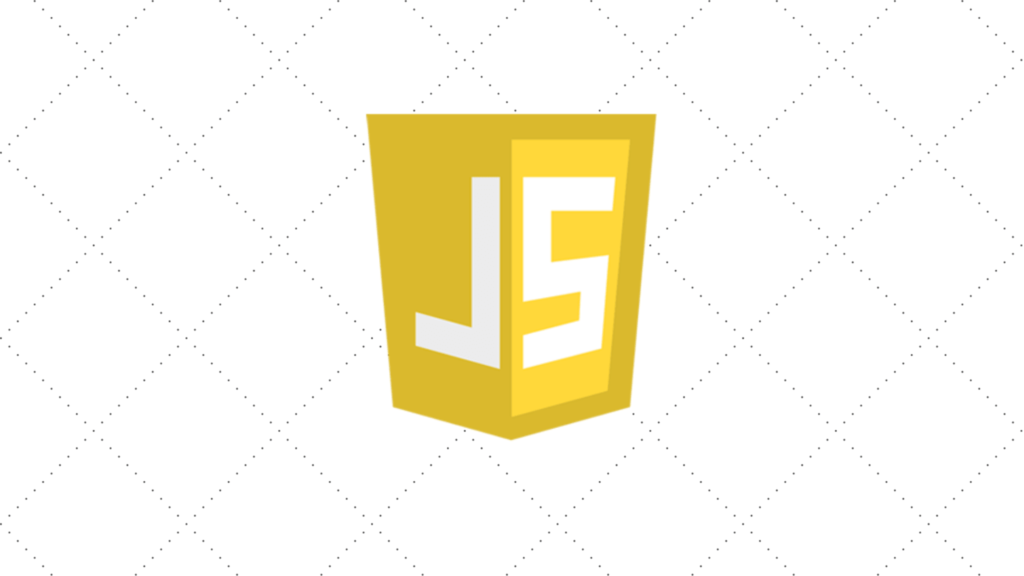
3/ Setting Up Capybara for JavaScript Interaction
Setting Up Capybara for JavaScript
For effective JavaScript alert, confirm, and prompt testing with Capybara, proper setup is key.
First, add the capybara
gem to your Gemfile:
Ruby
gem 'capybara'
In Rails applications, include capybara/rails
in your test helper file:
Ruby
require 'capybara/rails'
JavaScript testing requires a driver supporting JavaScript execution. Capybara offers options like Selenium WebDriver for real browsers like Firefox and Chrome. Add the selenium-webdriver
gem to your Gemfile:
Ruby
gem 'selenium-webdriver'
Run bundle install
to install these gems.
Next, configure Capybara to use your chosen JavaScript driver. For example, to use Selenium with Chrome, set the javascript_driver
option in your Capybara configuration file (e.g., env.rb
for Cucumber):
Ruby
Capybara.javascript_driver = :selenium_chrome
You can temporarily switch drivers for specific tests using metadata like js: true
in RSpec or tags like @javascript
in Cucumber.
With this setup, Capybara can interact with JavaScript dialog boxes. Use Capybara’s methods to handle these dialogs and verify expected application behavior.
Handling JavaScript Alerts
Use accept_alert
for alert()
functions. Here’s an example:
Ruby
accept_alert do
click_button('Show Alert')
end
expect(page).to have_content('Alert accepted')
accept_alert handles
the alert triggered by the button click. Assertions then verify the outcome.
Handling JavaScript Confirms
Use accept_confirm
for confirm()
functions. Here’s an example:
Ruby
accept_confirm do
click_button('Delete Item')
end
expect(page).to have_content('Item deleted')
accept_confirm handles the confirm. Use dismiss_confirm to simulate "Cancel":
Ruby
dismiss_confirm do
click_button('Delete Item')
end
expect(page).to have_content('Item not deleted')
These methods allow straightforward interaction with JavaScript confirms in Capybara tests, ensuring correct application behavior.
Handling JavaScript Prompts with Capybara
Capybara handles JavaScript prompts, dialog boxes requesting user input. The accept_prompt method allows interaction by providing input text and automatically clicking “OK.”
Here’s an example:
Ruby
accept_prompt(with: 'John Doe') do
click_button('Enter Name')
end
expect(page).to have_content('Hello, John Doe!')
accept_prompt
handles the prompt triggered by the button click. The with
option specifies the input text. The code within the block executes, and the prompt is accepted with the provided input. Assertions then verify the outcome.
This method allows your Capybara tests to interact with JavaScript prompts for comprehensive web application testing.
4/ Advanced Techniques for Handling Custom Modals
Capybara’s built-in methods handle standard JavaScript dialogs. However, custom modals (like those built with Bootstrap) require different interaction techniques due to their unique HTML structures.
Visibility and Accessibility
Before interaction, ensure the modal is visible and accessible in the DOM. Use has_css? to check for the modal’s container element:
Ruby
expect(page).to have_css('#myModal') # Check for modal with ID 'myModal'
Use within
to scope interactions to the modal’s container:
Ruby
within('#myModal') do
click_button('Close')
end
Interacting with Modal Elements
After confirming the modal’s presence, interact with its elements using Capybara’s standard methods: click_link
, click_button
, and fill_in
.
Ruby
within('#myModal') do
fill_in 'modalInput', with: 'Some text'
click_button 'Submit'
end
Waiting for Modal Actions
For asynchronous modal actions (like AJAX requests), use Capybara’s waiting capabilities. Use within
with a timeout or expect(page).to have_content(...)
with a wait time.
Example with Bootstrap Modal
Ruby
click_button 'Open Modal'
expect(page).to have_css('#myModal', wait: 5) # Wait for modal to appear
within('#myModal') do
click_button 'Close'
end
expect(page).not_to have_css('#myModal') # Assert modal is no longer visible
These techniques allow effective handling of custom modals in Capybara tests, ensuring comprehensive coverage of your web application’s JavaScript.
5/ Troubleshooting Common Issues
Capybara usually handles JavaScript dialogs well, but issues can arise. Here are some common problems and solutions:
Timing Issues
JavaScript dialogs are often asynchronous. If your test interacts with a dialog before it loads, it might fail. Use Capybara’s waiting mechanisms, such as within
with a timeout or have_css?
with a wait
option, to ensure Capybara waits for the dialog.
Incorrect Driver
The default :rack_test
driver doesn’t support JavaScript. For JavaScript dialogs, use a capable driver like :selenium_chrome
or :selenium_headless
. Switch drivers temporarily for specific tests using metadata or tags.
Modal Issues
For custom modals, ensure they are visible and accessible in the DOM before interaction. Use has_css?
to check for the modal’s presence and within
to scope interactions.
Debugging with execute_script
Use execute_script
to inject JavaScript code for debugging. This allows you to manipulate the page, retrieve information, and gain insights during the test.
Interactive Debugging with Pry
Use tools like Pry to pause test execution, inspect the browser state and variables, and step through your code. This helps pinpoint errors.
Overriding Alerts
In specific cases, you can override the alert
, confirm
, or prompt
functions in JavaScript using evaluate_script
to consistently accept or dismiss dialogs. Use this cautiously, as it might mask issues in your application’s alert handling.
Understanding these issues and using appropriate debugging techniques will help you effectively handle JavaScript dialogs in Capybara tests.
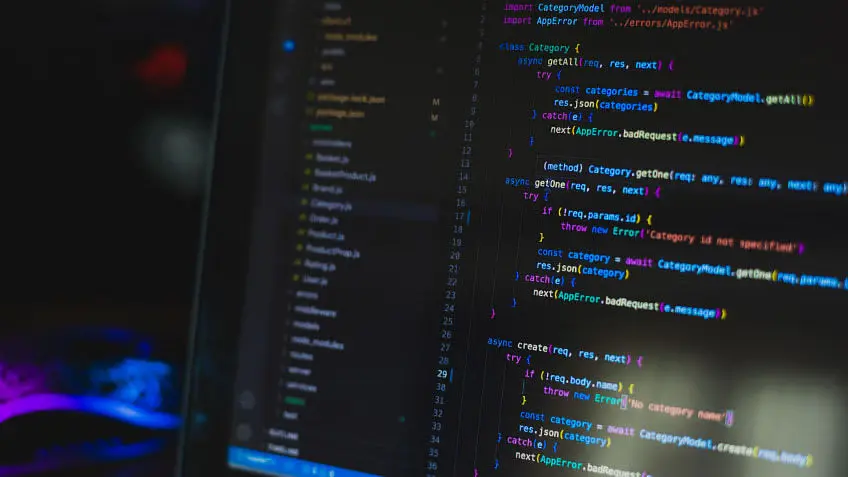
Conclusion
In this blog post, we explored the significance of handling JavaScript alerts, confirms, and prompts in Capybara tests for web applications. As one of the leading IT solutions, including those relevant to Ruby on Rails, Vinova delved into the role of JavaScript dialog boxes in user interactions and how Capybara facilitates seamless interaction with these elements. By understanding the setup process, utilizing Capybara’s helper methods, and employing advanced techniques for custom modals, you can ensure comprehensive testing of your web application’s JavaScript functionality, leading to a more robust and user-friendly experience.
Stay in the know and drop by our blog regularly to keep up with the latest insights, trends, and expert advice. Subscribe to Vinova’s newsletter to receive updates straight to your inbox and never miss a beat from one of the leading IT solutions in Singapore.