ActiveRecord, the ORM (Object-Relational Mapping) system in Ruby on Rails, is a powerful tool that simplifies database interactions, especially for developers maintaining legacy systems or working on projects that still use older versions of Rails. As Rails developers, a solid understanding of ActiveRecord’s update methods is crucial for efficient and reliable data manipulation. While seemingly straightforward, choosing the right update method can significantly impact your application’s performance and data integrity.
In this post, we’ll delve into the nuances of `update
`, `update_attribute
`, and other related methods, exploring their differences, use cases, and best practices. Whether you’re a seasoned Rails veteran or just starting your journey, mastering these methods will empower you to write cleaner, more efficient code.
Table of Contents
1. ActiveRecord Update Methods
1.1 Overview of ActiveRecord
ActiveRecord is the ORM (Object-Relational Mapping) system in Rails. It allows you to interact with your database using Ruby objects, abstracting away the complexities of SQL queries. This means you can perform CRUD (Create, Read, Update, Delete) operations on your database using an object-oriented approach. ActiveRecord automatically creates methods for accessing and manipulating data based on the columns in your database tables.
1.2 Different Update Methods in ActiveRecord
ActiveRecord provides a variety of methods for updating records in your database. Here’s a breakdown of some common ones:
update(id, attributes)
: This method updates an object (or multiple objects) with the given attributes, but only if validations pass. It returns the updated object regardless of whether the save was successful. You can use it to update a single record or multiple records. For example, to update a single record:
RubyPerson.update(15, user_name: 'Samuel', group: 'expert')
To update multiple records:
Rubypeople = { 1 => { "first_name" => "David" }, 2 => { "first_name" => "Jeremy" } }
Person.update(people.keys, people.values)
When updating a large number of records, using update can lead to performance issues as it executes an UPDATE
query for each record.
update_attribute(name, value):
This method updates a single attribute and saves the record without performing validations. It’s useful for boolean flags or situations where validations need to be bypassed. However, it still invokes callbacks and updates theupdated_at
timestamp. For example:
Rubyuser = User.last
user.update_attribute(:status, "active")
update_attributes(attributes)
: This method updates all attributes from a provided hash and saves the record, but only if validations pass. It’s similar toupdate
, but it takes a hash of attributes instead of separate arguments. This method has been deprecated in Rails 6.1 in favor of update. For example:
Rubyuser = User.last
user.update_attributes(first_name: 'update name', status: "active")
update_column(name, value)
: This method updates a single attribute directly in the database, bypassing validations and callbacks. It doesn’t update theupdated_at
timestamp. For example:
Rubyuser = User.last
user.update_column(:status, "inactive")
update_columns(attributes):
This method is similar toupdate_column
, but allows updating multiple attributes in a single call. For example:
Rubyuser = User.last
user.update_columns(first_name: 'new name', status: "inactive")
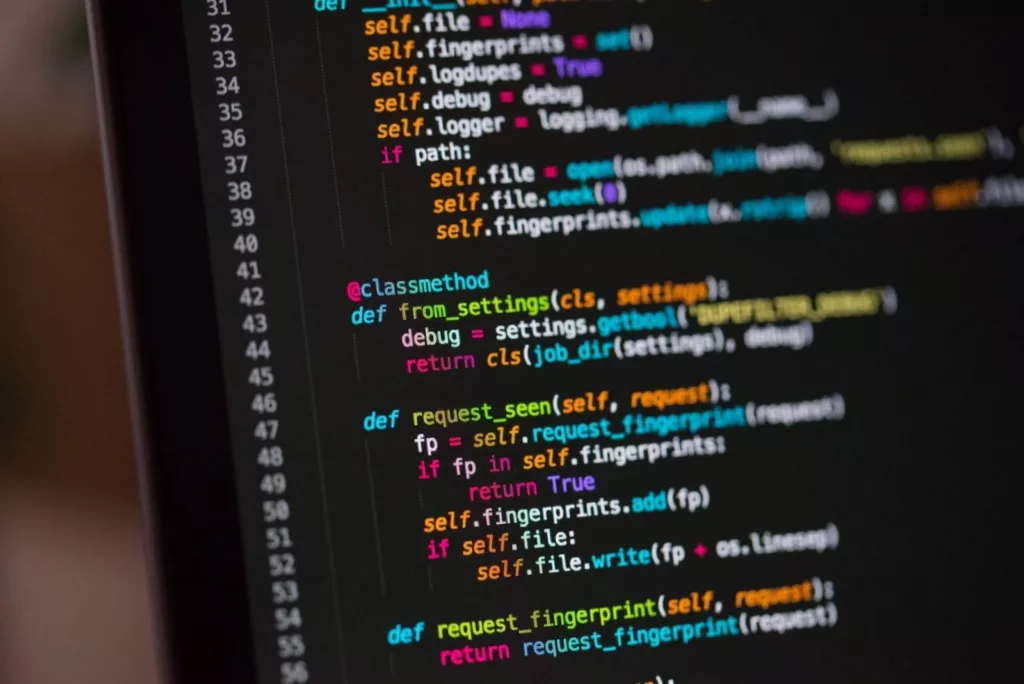
Comparison of These Methods
Here’s a table summarizing the key differences between these methods:
Method | Validations | Callbacks | updated_at Updated |
update | Performed | Invoked | Yes |
update_attribute | Skipped | Invoked | Yes |
update_attributes | Performed | Invoked | Yes |
update_column | Skipped | Skipped | No |
update_columns | Skipped | Skipped | No |
Export to Sheets
It’s important to choose the right update method based on your specific needs and the context of your application. If you need to ensure data integrity, using update
with proper validations is recommended. If you need to bypass validations for a specific reason, update_attribute
can be used. And if you need to perform a bulk update or need the fastest way to update attributes, update_all
, update_column
, or update_columns
might be suitable options.
2. update_attribute vs. update_attributes
2.1 update_attribute
- Description and Usage: This method updates a single attribute of an ActiveRecord object and saves the change to the database. It bypasses validations but still invokes callbacks and updates the
updated_at
timestamp . It’s useful for situations where you need to quickly update a single attribute without triggering validations, such as toggling a boolean flag or updating a counter .
Example Code Snippet:
Rubyuser = User.find(1)
user.update_attribute(:status, 'active')
# Updates the status attribute to ‘active’ without validations
- Pros:
- Skips validations, allowing for faster updates when validations are not necessary.
- Invokes callbacks, enabling you to perform actions related to the attribute update.
- Updates the
updated_at
timestamp, maintaining consistency in your data.
- Cons:
- Bypasses validations, which can lead to data integrity issues if not used carefully.
- Only updates a single attribute at a time, which can be inefficient for multiple attribute updates.
2.2 update_attributes
- Description and Usage: This method updates multiple attributes of an ActiveRecord object from a provided hash. It performs validations before saving the changes to the database and invokes callbacks . It’s similar to the update method but takes a hash of attributes instead of separate arguments .
Example Code Snippet:
Rubyuser = User.find(1)
user.update_attributes(first_name: 'John', last_name: 'Doe', email: '[email protected]')
- Pros:
- Updates multiple attributes in a single call, which can be more efficient than updating individual attributes.
- Performs validations, ensuring data integrity.
- Invokes callbacks, allowing for related actions to be performed.
- Cons:
- Can be slower than
update_attribute
due to validations. - Deprecated in Rails 6.1 in favor of update .
- Can be slower than
2.3 Deprecation of update_attributes
in Rails 6 and later
The update_attributes
method was deprecated in Rails 6.1 and is scheduled to be removed in Rails 7.1 . This deprecation was introduced to align with other Active Record methods like create and provide better semantics when updating a resource .
Transition to using update:
To update your code, simply replace update_attributes
with update
. The update method provides the same functionality as update_attributes
while adhering to the latest Rails conventions
For example:
Ruby
# Rails 6.0 and earlier
user.update_attributes(first_name: 'John', last_name: 'Doe')
# Rails 6.1 and later
user.update(first_name: 'John', last_name: 'Doe')
By transitioning to update, you ensure your code is compatible with the latest Rails versions and follows best practices.
3. Bypassing Validations in Rails
3.1 Explanation of Validations in Rails
Validations are an essential part of any Rails application, ensuring that only valid data is saved into your database . They act as gatekeepers, preventing invalid data from being persisted and maintaining data integrity . Validations are defined in your models and are automatically run before creating or updating records . If any validation fails, the object is marked as invalid, and the save operation is aborted . This prevents incorrect or inconsistent data from entering your database, which could lead to application errors or unexpected behavior.
3.2 Methods for Bypassing Validations
While validations are crucial for data integrity, there are situations where you might need to bypass them. Rails provides several methods for this, each with its own use cases and considerations:
update_attribute(name, value)
: This method updates a single attribute and saves the record without performing validations . It’s useful for boolean flags or situations where validations need to be bypassed . However, it still invokes callbacks and updates theupdated_at
timestamp .update_column(name, value)
: This method updates a single attribute directly in the database, bypassing both validations and callbacks . It doesn’t update the updated_at timestamp . This is useful when you need to update an attribute without triggering any side effects, such as when performing database migrations or bulk updates.update_columns(attributes)
: This method is similar toupdate_column
, but allows updating multiple attributes in a single call . It also bypasses validations and callbacks and doesn’t update the updated_at timestamp.
Use Cases and Considerations
Bypassing validations should be done with caution, as it can compromise data integrity if not handled carefully . Here are some use cases where bypassing validations might be necessary:
- Updating a counter: When incrementing or decrementing a counter, you might not want to run validations on other attributes.
- Setting a flag: When toggling a boolean flag, validations might not be relevant.
- Mass updates: When performing bulk updates, running validations for each record can be inefficient.
- Migrations: When migrating data, you might need to bypass validations to import data that doesn’t meet current validation rules.
When bypassing validations, it’s essential to understand the potential risks and take appropriate measures to mitigate them. Consider using database constraints or transactions to provide an extra layer of protection when validations are bypassed . Always carefully consider the implications of bypassing validations and ensure that data integrity is maintained through alternative means when necessary.
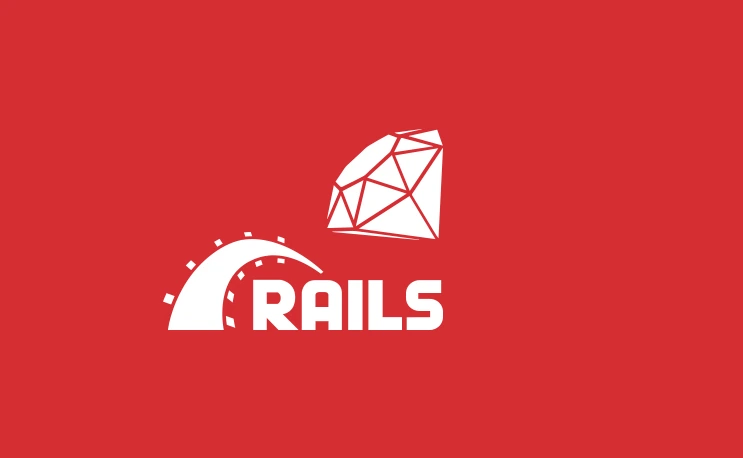
4. Rails Callbacks and Validations
4.1 Overview of Rails Callbacks
Callbacks are methods that get called at specific moments of an object’s life cycle . With callbacks, it is possible to write code that will run whenever an Active Record object is created, saved, updated, deleted, validated, or loaded from the database . This allows you to trigger logic before or after an alteration of an object’s state .
Before and After Callbacks
Callbacks are typically categorized as “before” or “after” callbacks, indicating when they are executed relative to a specific event. For example, a before_save
callback will run before a record is saved to the database, while an after_save
callback will run after the record has been saved .
Example Scenarios
Here are a few examples of how callbacks can be used in Rails applications:
- Updating a timestamp: You can use a
before_save
callback to update a timestamp when a user’s profile gets modified . - Sending notifications: An
after_create
callback can be used to send a welcome email to a new user . - Cleaning up associated records: An
after_destroy
callback can be used to delete associated records when a user is destroyed .
4.2 Interaction between Update Methods and Callbacks
Different update methods in Active Record interact with callbacks in different ways. Here’s how update_attribute
and update interact with callbacks:
How update_attribute
Interacts with Callbacks
The update_attribute
method skips validations but still invokes callbacks . This means that any callbacks associated with the save or update events will be triggered, even though validations are bypassed . This can be useful when you need to update an attribute without triggering validations but still want to perform actions related to the attribute update.
How update Interacts with Callbacks
The update method performs validations and invokes callbacks . This means that validations will be run before any callbacks are triggered, ensuring that only valid data is saved to the database . If any validation fails, the save operation is aborted, and callbacks are not executed . This ensures data integrity and consistency in your application.
Understanding the interaction between update methods and callbacks is crucial for building robust and reliable Rails applications. By carefully choosing the appropriate update method and defining relevant callbacks, you can ensure that your application behaves as expected and maintains data integrity.
5. Practical Examples and Use Cases
5.1 When to use update_attribute
The update_attribute
method is best suited for situations where you need to update a single attribute without triggering validations, while still invoking callbacks and updating the updated_at
timestamp. Here are some scenarios and examples:
- Toggling a boolean flag: Imagine you have a User model with a verified attribute (boolean) to indicate whether the user’s email address has been verified. You can use
update_attribute
to toggle this flag without triggering any other validations:
Rubyuser = User.find(1)
user.update_attribute(:verified, true)
- Updating a counter: If you have a Post model with a
views_count
attribute to track the number of times a post has been viewed, you can useupdate_attribute
to increment the counter without running validations on other attributes:
Rubypost = Post.find(1)
post.update_attribute(:views_count, post.views_count + 1)
- Updating an attribute in a
before_save
callback: In some cases, you might need to update an attribute based on the value of another attribute that is being updated. You can useupdate_attribute
inside abefore_save
callback to achieve this:
Rubyclass Order < ActiveRecord::Base
before_save :update_discount
-
private
def update_discount
if amount > 100
update_attribute(:discount, 0.1)
- end
-
end
end
5.2 When to use update
The update method is the preferred way to update ActiveRecord objects in most scenarios. It performs validations, invokes callbacks, and updates the updated_at
timestamp. Here are some scenarios and examples:
- Updating user profile information: When a user updates their profile, you typically want to validate the input to ensure data integrity. You can use update to achieve this:
Rubyuser = User.find(1)
user.update(first_name: 'John', last_name: 'Doe', email: '[email protected]')
- Updating an object with nested attributes: When dealing with nested attributes, update can handle the updates for both the parent and child objects while performing validations:
Rubyclass Order < ActiveRecord::Base
- has_many :line_items
accepts_nested_attributes_for :line_items
end
order = Order.find(1)
order.update(order_params) # order_params includes nested attributes for line_items
- Updating multiple records with different attributes: You can use update to update multiple records with different attributes in a single call:
Rubypeople = { 1 => { "first_name" => "David" }, 2 => { "first_name" => "Jeremy" } }
Person.update(people.keys, people.values)
Remember to choose the appropriate update method based on your specific needs and the context of your application. If you need to ensure data integrity, update is generally recommended. If you need to bypass validations for a specific reason, update_attribute
can be used with caution.
Conclusion
In this post, we’ve explored the nuances of ActiveRecord’s update methods, particularly `update
`, `update_attribute
`, and their related functionalities. We delved into the differences between these methods, focusing on their impact on validations, callbacks, and timestamp updates. Key takeaways include understanding when to leverage `update_attribute
` for specific, validation-bypassing updates, and when `update` is the more suitable choice for maintaining data integrity through validations.
For developers, it’s crucial to select the appropriate update method based on the specific requirements of your use case. Always prioritize data integrity by using `update
` with validations whenever possible. If bypassing validations is necessary, proceed with caution using `update_attribute
`, and be sure to implement alternative measures to ensure data consistency. Remember that `update_attributes
` is deprecated in Rails 6.1 and beyond; make the switch to `update
` to stay current with best practices.