Ruby is known for its elegance and flexibility, but to truly master this language, you need to go beyond the basics. Even in 2025, Ruby remains a popular choice among developers, with over 800,000 active developers worldwide. Additionally, Ruby on Rails powers over 1 million websites, including major platforms like GitHub, Shopify, and Airbnb. To stay competitive and create high-performance applications, it’s essential to dive deep into Ruby’s advanced features and best practices.
As one of the leading IT solutions in Singapore, Ruby on Rails is one of our top priorities. This article explores some must-know Ruby tricks that can significantly improve your code’s readability, efficiency, and maintainability.
Table of Contents
1. Utilize the Power of Metaprogramming
Metaprogramming, a technique where code operates on other code, is a cornerstone of Ruby’s flexibility. It allows for dynamic code generation and modification, leading to more concise, adaptable, and reusable code. With metaprogramming, you can reduce code duplication, improve flexibility, and even create domain-specific languages (DSLs). Ruby excels in metaprogramming, offering a variety of powerful tools. Let’s explore some of these tools:
1.1 send Method
The send method allows you to invoke a method by name programmatically. This is useful when you need to call methods dynamically based on user input or configuration.
Ruby
class MyClass
def greet(name)
puts "Hello, #{name}!"
end
end
obj = MyClass.new
obj.send(:greet, "World") # Output: Hello, World!
1.2 define_method Method
define_method enables dynamic method creation during runtime. This is particularly helpful when you need to define methods based on patterns or data.
Ruby
class MyClass
["eat", "walk"].each do |action|
define_method(action) do
puts "I am #{action}ing."
end
end
end
obj = MyClass.new
obj.eat # Output: I am eating.
obj.walk # Output: I am walking.
1.3 method_missing Method
The method_missing method is invoked when an object receives a message it cannot handle. This allows you to define dynamic behavior for undefined methods.
Ruby
class MyClass
def method_missing(method_name, *args, &block)
if method_name.to_s.include?("can_")
puts "I can #{method_name.to_s.sub("can_", "")}!"
else
super
end
end
end
obj = MyClass.new
obj.can_fly # Output: I can fly!
obj.can_swim # Output: I can swim!
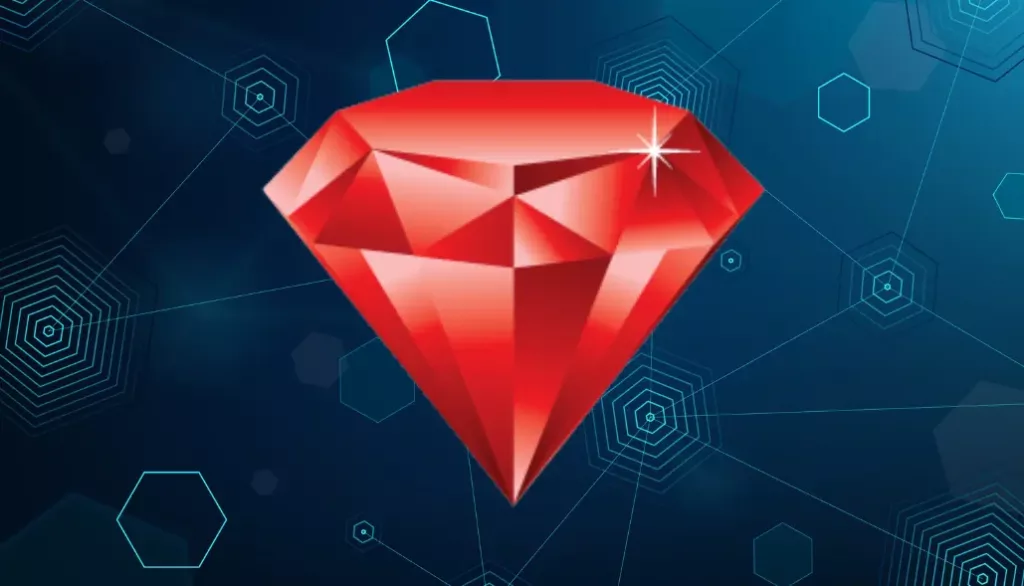
2. Embrace Ruby’s Lesser-Known Features
Ruby is packed with lesser-known features and methods that can simplify your code and improve its efficiency. These features often contribute to more idiomatic, efficient, and expressive Ruby code, allowing you to write code that is both concise and readable.
2.1. Working with Variables and Symbols
- Symbols: Symbols are immutable, memory-efficient alternatives to strings, often used as identifiers. They are particularly useful as keys in hashes.
Ruby
user = { :name => “John”, :age => 30 }
- Variable Prefixes: Ruby uses prefixes to denote the scope of variables. @ indicates instance variables, which are accessible within a specific instance of a class. @@ denotes class variables, shared across all instances of a class. $ signifies global variables, accessible throughout the entire program.
Ruby
class MyClass
@@count = 0 # Class variable
def initialize
@name = "Instance" # Instance variable
end
end
2.2. Useful Methods for Working with Collections
- tap Method: The tap method yields an object to a block and returns the object itself. This is useful for chaining methods and avoiding temporary variables, leading to more concise and readable code.
Ruby
user = User.new.tap do |u|
u.name = "Jane"
u.email = "[email protected]"
end
- bsearch Method: The bsearch method performs a binary search on a sorted array, providing efficient lookup with O(log n) complexity. This is significantly faster than linear search methods for large datasets.
Ruby
data = (1..100).to_a
data.bsearch { |x| x > 50 } # Returns 51
to_h
Method: This method allows you to convert an array of two-element arrays into a hash. It interprets each two-element array as a key-value pair, making it a convenient way to create hashes from structured data.
Ruby
data = [[:name, "John"], [:age, 30]]
hash = data.to_h # Returns { :name => "John", :age => 30 }
2.3. Conditional Logic and Operators
- Flip-Flop Operator: The flip-flop operator (.. or …) provides a concise way to simplify conditional logic when dealing with ranges. It allows you to define a condition that remains true within a specific range, making code more readable and efficient.
Ruby
lines.each do |line|
if line =~ %r{^\s*/\*} .. line =~ %r{^\s*\*/}
# Code to execute while inside a multi-line comment
else
# Code to execute outside the comment block
end
end
- Unary Methods: Unary methods are prefixed method calls on particular objects within Ruby. They often provide shorthand operations or allow you to override existing behavior. For example, you can override the unary minus (-) method for an array to clear its contents9.
Ruby
class Array
def -@
clear
end
end
arr = [1, 2, 3]
-arr # Clears the array
2.4. Safe Navigation Operator (&.)
The safe navigation operator (&.) allows you to call methods on an object without raising an exception if the object is nil. This is particularly useful when dealing with potentially nil objects, such as optional attributes in a data structure.
Ruby
user&.name # Returns user.name if user is not nil, otherwise returns nil
It’s also beneficial when working with hashes, as it allows you to access keys that may not exist without causing errors.
Ruby
hash&.dig(:key1, :key2) # Safely accesses nested keys in a hash
3. Write Readable and Maintainable Code
Writing clean, readable code is crucial for maintainability and collaboration. Ruby offers several features and best practices to achieve this.
3.1. Follow Style Guides
Adhering to style guides, such as the Ruby Style Guide, ensures consistency and readability across your codebase. Style guides provide conventions for formatting, naming, and code structure, making it easier for developers to understand and work with the code. Use tools like RuboCop to automatically enforce these guidelines and maintain a consistent code style.
3.2. Keep It Concise
Favor concise code by using Ruby idioms and avoiding unnecessary verbosity. Ruby offers a variety of features and idioms that allow you to express complex logic in a clear and concise manner. For example, conditional assignments and ternary operators can simplify conditional statements.
Ruby
# Concise conditional assignment
result = condition ? value1 : value2
3.3. Avoid Excessive Method Chaining
While method chaining can be useful for conciseness, excessive chaining can hinder readability. Long chains of methods can be difficult to follow and understand, especially when dealing with complex logic8. Consider extracting logic into separate methods or classes to improve readability and maintainability.
Ruby
# Instead of:
result = object.method1.method2.method3
# Consider:
intermediate_result = object.method1.method2
result = intermediate_result.method3
3.4. Use Meaningful Names
Choose descriptive names for variables, methods, and classes to convey their purpose and improve code understanding. Meaningful names make it easier to grasp the intent of the code and reduce the need for comments.
3.5. Write Tests
Automated testing is essential for ensuring code quality and preventing regressions. Use testing frameworks like RSpec to write comprehensive tests that cover different aspects of your code. Tests act as a safety net, allowing you to make changes with confidence and catch potential issues early on.
3.6. Refactor Regularly
Refactor your code regularly to improve its structure, reduce duplication, and address technical debt. Refactoring involves restructuring existing code without changing its external behavior. It helps keep your codebase clean, maintainable, and adaptable to future changes.
4. Optimize for Performance
While Ruby prioritizes developer happiness, performance optimization is crucial for ensuring applications are responsive and efficient, especially as they scale.
4.1. Choose the Right Data Structures
Select appropriate data structures based on your needs. Arrays are efficient for indexed access, while hashes excel at key-value lookups. Choosing the right data structure can significantly impact the performance of your code, especially when dealing with large datasets.
4.2. Avoid Unnecessary Object Creation
Minimize object creation to reduce memory consumption and garbage collection overhead. Use string interpolation and enumerators to avoid unnecessary object creation. Excessive object creation can lead to performance bottlenecks, especially in memory-intensive applications.
4.3. Use Memoization
Memoization caches the results of expensive computations, preventing redundant calculations and improving performance. This technique is particularly useful for functions with repetitive calculations or expensive operations.
Ruby
def fibonacci(n, memo = {})
return memo if memo
return n if n < 2
memo = fibonacci(n - 1, memo) + fibonacci(n - 2, memo)
end
4.4. Use Symbols in Hashes
When defining hashes, prefer symbols over strings for keys. Symbols are more efficient for hash lookups due to their immutability and internal representation.
4.5. Profile Your Code
Use profiling tools like ruby-prof
and stackprof
to identify performance bottlenecks and optimize critical sections of your code. Profiling helps you understand where your application spends most of its time, allowing you to focus your optimization efforts on the most impactful areas.
4.6. Use an Application Performance Monitoring (APM) Tool
An APM tool can be invaluable for monitoring and improving the performance of your Ruby application. It provides insights into response times, database queries, and other performance metrics, helping you identify and address bottlenecks.
4.7. Choose the Right Ruby Implementation
Consider using a faster Ruby implementation, such as JRuby
, Rubinius
, or TruffleRuby
, if you require further performance improvements. These implementations can offer significant performance gains, especially for CPU-intensive workloads.
5. Avoid Common Pitfalls
These pitfalls can lead to subtle bugs, unexpected behavior, and performance issues that can be difficult to debug. Being aware of them can help you write more robust and efficient Ruby code.
5.1. Operator-Related Pitfalls
- Operator Precedence: Understand the precedence of operators like and, or, and not to avoid unexpected results. These operators have lower precedence than their symbolic counterparts (
&&
,||
,!
), which can lead to subtle bugs if not used carefully. - Global Variables: Avoid using global variables whenever possible. They can make code harder to maintain, debug, and test due to their widespread accessibility and potential for unintended side effects.
5.2. Method-Related Pitfalls
- Equality Comparisons: Differentiate between
equal?
,eql?
,===
, and==
for accurate equality comparisons.equal?
checks for object identity,eql?
for hash key equality, === for case equality, and==
for generic equality. super vs super()
: Usesuper
to call the parent method with the same arguments, andsuper()
to call it without arguments. Using the wrong form of super can lead to unexpected behavior and difficult-to-trace bugs.- Hash Initialization: Be mindful of the differences between
Hash.new()
andHash.new { |h, k| h = }
when initializing hashes with default values. The former uses the same default value for all keys, while the latter creates a new default value for each key. map
vsmap!
: When modifying arrays, usemap!
for in-place modification instead ofmap
. Usingmap
creates a new array, which can be less efficient for large datasets.- Rescuing
StandardError
: Avoid rescuingStandardError
unless absolutely necessary. Rescuing this general exception can mask unexpected errors and make debugging more difficult. Instead, rescue specific exceptions to handle known error conditions.
5.3. String-Related Pitfalls
- Immutable Strings: Use
# frozen_string_literal: true
at the beginning of your files or enable the--enable-frozen-string-literal
flag to make all literal strings immutable. This can improve performance and prevent unintended modifications. It can also affect string comparisons, as immutable strings with the same content will be considered the same object. - length vs size: While both methods often return the same result, length is generally preferred for arrays and strings, while size is more common for hashes and other collections.
Conclusion
Mastering Ruby involves more than just understanding its syntax. By embracing metaprogramming, exploring lesser-known features, writing clean code, optimizing for performance, and avoiding common pitfalls, you can elevate your Ruby skills significantly. These techniques not only improve the efficiency and maintainability of your code but also allow you to harness the full power of Ruby’s flexibility and elegance. As you continue your Ruby journey, remember that continuous learning and experimentation are key to unlocking even greater proficiency in this versatile language.