Progressive Web Apps (PWAs) offer compelling advantages for US businesses in 2025, promising app-like engagement from an efficient single codebase. However, realizing this potential hinges on overcoming significant cross-browser compatibility challenges, especially limitations impacting the large US iOS user base.
This report detailed the crucial strategies required: fundamental progressive enhancement, rigorous multi-platform testing, and smart tooling (like Workbox). Applying these techniques enables developers to navigate complexities and build reliable, effective PWAs that deliver on their promise—ensuring both broad reach with a functional baseline and a rich user experience where modern browser features are supported.
Table of Contents
Understanding Progressive Web Apps (PWAs)
Looking for app-like user engagement without managing separate native codebases? Progressive Web Apps (PWAs) offer a solution. Built with standard web technologies (HTML/CSS/JS) and enhanced APIs, PWAs deliver features like offline access and installability directly from a browser.
The “Progressive” element ensures a core experience functions universally, while advanced features activate only on capable devices. This maximizes reach across the diverse US market. Businesses implementing PWAs often report noticeable increases in user engagement metrics, bridging the gap between web reach and native app interaction.
Why Cross-Browser Compatibility Is A Challenge In PWA Development
While Progressive Web Apps promise broader reach from a single codebase, achieving consistent performance across browsers presents technical hurdles. Why does a PWA sometimes look or behave differently in Chrome versus Safari or Firefox?
The core issue lies in the distinct engines browsers use:
- Rendering Engines: Blink (Chrome, Edge), Gecko (Firefox), and WebKit (Safari) interpret HTML/CSS differently.
- JavaScript Engines: V8 (Chrome, Edge), SpiderMonkey (Firefox), and JavaScriptCore (Safari) execute code with potential variations.
Vendors (Google, Apple, Mozilla) also implement new web standards and PWA-specific APIs, like Service Workers or Background Sync, at different paces. This leads to compatibility challenges:
- CSS & Layout: Visual consistency requires careful testing. Modern features like Flexbox or Grid might have subtle cross-browser differences, and older CSS may need vendor prefixes or resets for a uniform baseline.
- JavaScript & APIs: Modern JavaScript (ES6+) often needs transpilers (like Babel) and polyfills for older browser support. Crucially, the availability and behavior of PWA APIs can vary; for instance, Background Sync support isn’t universal.
- PWA Feature Implementation: The user experience for core PWA features differs. Installation prompts are proactive on Android/Chromium but require manual steps via the Share menu on iOS/Safari, impacting install rates significantly, especially given Safari’s substantial mobile browser share in the US. Offline caching and push notification behavior can also diverge.
- Rendering Nuances: Minor visual discrepancies in fonts or image scaling can occur. Using the correct <!DOCTYPE> prevents browsers from entering “Quirks Mode,” which causes major layout issues.
Developers must plan for graceful degradation, ensuring a functional baseline on older browsers lacking full PWA support.
Ultimately, browser updates, evolving standards (like those pursued by Project Fugu), and new devices make compatibility an ongoing effort demanding continuous testing and adaptation, not just a one-time check.
Browser Support Landscape for PWA Features
Before committing to specific PWA functionalities, verifying browser support is crucial. Which features work reliably across the browsers your US audience uses most?
Support levels for core PWA technologies differ across Chrome, Firefox, Edge, and Safari on desktop and mobile. Always consult up-to-date resources like caniuse.com and MDN Web Docs for detailed compatibility tables, focusing on the latest stable browser versions.
Here’s a snapshot of support for common PWA APIs:
- Service Workers: The foundation for offline use and push notifications enjoys broad support in current versions of Chrome, Edge, Firefox, and Safari. Legacy browsers like Internet Explorer lack support.
- Web App Manifest & Installability: While most modern browsers parse the manifest file, the ability to “install” the PWA (Add to Home Screen – A2HS) varies:
- Chromium (Chrome, Edge): Robust support on desktop/Android, often with proactive install prompts.
- Firefox: Supports installation on Android, but not desktop.
- Safari (iOS/Desktop): No automatic prompts. Installation on iOS requires manual user steps via the Share menu, a significant consideration given Safari’s large US mobile market share. Desktop Safari does not support manifest-based installation.
- Push API (Notifications): Widely supported on Chromium browsers and Firefox. Safari support has nuances:
- Desktop: Supported from Safari 16.1 onwards.
- iOS: Supported from iOS 16.4, but only after the PWA is manually added to the Home Screen, and user interaction is often needed for permission.
- Background Sync API (One-off): Allows deferring actions until connectivity returns. Primarily supported only in Chromium browsers (Chrome, Edge). Firefox and Safari do not support it.
- Periodic Background Sync API: Enables scheduled background tasks. Support is even more limited, generally restricted to installed PWAs on recent Chromium versions (e.g., Chrome 80+). Firefox and Safari lack support.
Understanding these differences helps prioritize features and set realistic expectations for your PWA’s cross-browser behavior.
The following table summarizes the general support status for key PWA features across major browsers as of the time of this report.
PWA Feature Browser Support Summary
Feature | Chrome (Desktop/Android) | Edge (Desktop/Android) | Firefox (Desktop) | Firefox (Android) | Safari (Desktop) | Safari (iOS) |
Service Worker (Core) | Yes | Yes | Yes | Yes | Yes | Yes |
Web App Manifest | Yes | Yes | Yes | Yes | Yes | Yes |
Installability (A2HS) | Yes (Prompt) | Yes (Prompt) | No | Yes (Manual) | No | Yes (Manual, Safari only) |
Push API | Yes | Yes | Yes | Yes | Yes (v18+) | Yes (iOS 16.4+, Limited) |
Background Sync | Yes | Yes | No | No | No | No |
Periodic Bg. Sync | Yes (Limited) | Yes (Limited) | No | No | No | No |
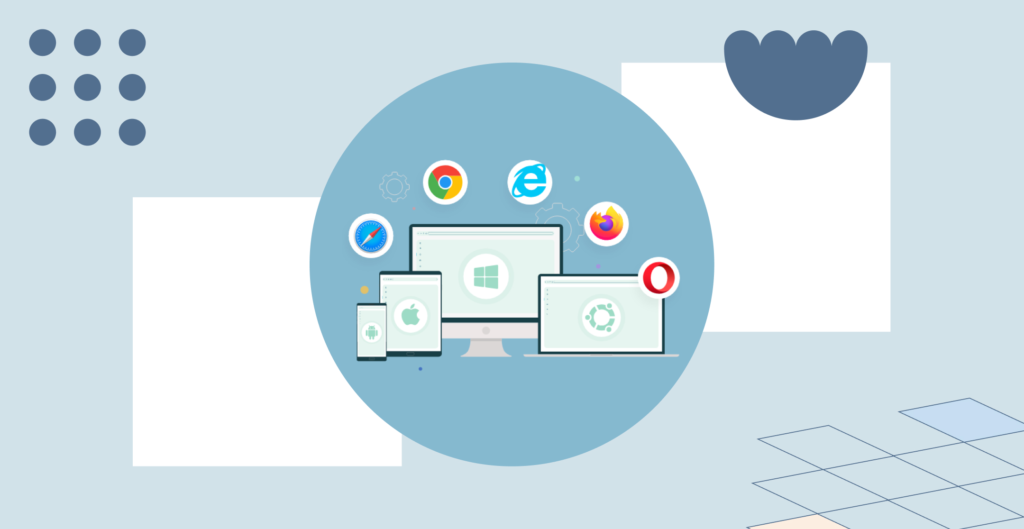
Best Practices for Achieving Cross-Browser Compatibility
How do you ensure your PWA delivers a solid experience when faced with differing browser capabilities across the US market? Relying solely on testing isn’t enough; proactive development strategies are needed.
Building for the wide array of browser versions and devices requires a structured approach. To create PWAs that function reliably despite browser variations, integrate these core practices into your workflow: progressive enhancement, feature detection, graceful degradation, responsive design, and continuous performance optimization. These methods help manage inconsistencies and aim for the best possible user experience on any given device.
Progressive Enhancement (PE)
How can you ensure your PWA provides value to every user, regardless of their browser or device capabilities in the diverse US market? Progressive Enhancement offers a robust strategy.
This approach involves building your application in layers, starting with a universal foundation:
- Core Content & Functionality: Use semantic HTML for the essential experience. Basic HTML elements are understood by virtually 100% of browsers, guaranteeing a functional baseline for everyone.
- Presentation: Apply CSS to enhance visual styling and layout for browsers that correctly interpret it.
- Behavior: Layer JavaScript for interactivity, client-side validation, and richer features where supported.
- Advanced Features: Implement PWA-specific APIs (like service workers for offline access or push notifications) as final enhancements for capable browsers.
Consider a simple HTML form: it works natively. PE adds JavaScript for client-side checks or AJAX submission in modern browsers, enhancing the experience without breaking basic functionality for users on older browsers.
This method ensures a working baseline everywhere and makes the application more resilient to future browser changes. It contrasts with Graceful Degradation (which starts complex and removes features) and directly embodies the “progressive” aspect of PWAs.
Feature Detection
You’ve designed enhanced PWA features, but how do you implement them safely across the varied browser landscape in the US without causing errors for users whose browsers lack support? The answer lies in Feature Detection.
Feature detection involves programmatically checking if the user’s current browser supports a specific capability before attempting to use it. This practice is fundamental for implementing Progressive Enhancement correctly, preventing JavaScript errors or broken layouts when APIs or CSS properties are missing.
Common JavaScript techniques include:
Checking for object properties/methods: Verifying if an expected feature exists.
JavaScript
if (‘serviceWorker’ in navigator) { /* Use Service Worker */ }
if (‘SyncManager’ in window) { /* Use Background Sync */ }
Testing properties on created elements: Checking capabilities of specific HTML elements.
JavaScript
const supportsCanvas = !!document.createElement(‘canvas’).getContext;
Using window.matchMedia(): Testing for CSS media features like display modes.
- JavaScript
if (window.matchMedia(‘(display-mode: standalone)’).matches) { /* App is installed */ }
Using CSS.supports(): Directly querying CSS property support.
- JavaScript
if (CSS.supports(‘display’, ‘grid’)) { /* Use CSS Grid */ }
Using the Permissions API: Checking user permissions for sensitive APIs.
- JavaScript
const status = await navigator.permissions.query({name: ‘geolocation’});
CSS also offers native feature detection via the @supports rule, allowing you to apply styles conditionally based on browser support. By implementing these checks, you ensure enhanced features are only activated when available, maintaining a stable experience for all users.
Graceful Degradation (GD)
What’s the strategy when your PWA’s core value heavily relies on modern browser features, but you still need a plan for users on older systems, common across segments of the US market? This is where Graceful Degradation (GD) comes in.
GD operates opposite to Progressive Enhancement. You start by building the full-featured application assuming modern browser capabilities. Then, using feature detection, you identify unsupported features in less capable browsers and provide simpler fallbacks or alternative content. The goal is to prevent a completely broken site, ensuring a basic level of usability for nearly all users, even if the experience is simplified.
While often contrasted with PE, the approaches aren’t mutually exclusive. GD can be practical when:
- Adapting an existing, complex web application into a PWA.
- A modern feature is central, but a fallback is needed for broader reach.
For instance, a PWA might use complex WebGL animations for product visualization. With GD and feature detection, it could display static images or simpler CSS transitions on browsers lacking WebGL support, ensuring users can still view the product.
Responsive Design
Your PWA needs to perform flawlessly whether viewed on a desktop, tablet, or smartphone. With mobile devices accounting for roughly 60% of web traffic in the US as of early 2025, how do you ensure a seamless experience across such diverse screen sizes? Responsive Web Design principles are the solution.
Implementing responsive design is a baseline requirement for PWAs aiming for cross-device compatibility. Key techniques include:
- Fluid Grid Layouts: Utilizing relative units (percentages, viewport units) and flexible systems like CSS Grid or Flexbox allows layouts to adjust smoothly to different screen widths.
- Flexible Media: Ensuring images, videos, and other media automatically resize to fit their containers prevents content overflow or distortion.
- Media Queries: Applying specific CSS rules based on device characteristics (screen width, height, orientation, resolution) optimizes presentation and interaction for each context.
Applying these principles ensures your PWA content remains readable and interactive elements are easy to use, regardless of the device accessing it.
Performance Optimization
Users install PWAs expecting app-like speed and responsiveness, often higher than standard websites. How do you ensure your PWA delivers, especially considering varying network conditions across the US? Failing to optimize can lead to frustration; industry studies consistently show that even 1-3 second delays in load time significantly increase user bounce rates. Continuous performance optimization is therefore critical.
Focus on these key areas:
- Minimize Load Times: Implement code splitting to load JavaScript chunks on demand, lazy-load non-critical resources (like below-the-fold images), and always minify/compress assets (JS, CSS, images).
- Optimize Images: Utilize modern formats like WebP or AVIF, which can offer substantial file size reductions (often 25-50% or more) compared to older formats like JPEG/PNG, while maintaining quality. Use responsive image techniques (srcset) to serve appropriately sized files.
- Leverage Caching: Use service workers for smart caching. Implement the App Shell pattern to cache the core UI for near-instant perceived loading. Apply suitable caching strategies (e.g., cache-first, stale-while-revalidate) and consider patterns like PRPL for efficient resource loading.
- Measure & Monitor: Regularly audit performance using tools like Lighthouse, PageSpeed Insights, and browser DevTools to identify and address bottlenecks proactively.
Essential Tools, Libraries, and Polyfills for Cross-Browser PWA Development
Developing cross-browser compatible PWAs often involves leveraging specific tools and libraries to manage complexity, ensure compatibility, and streamline common tasks.
Service Worker Libraries: Workbox
Manually coding service worker logic for caching, offline support, and background tasks can be intricate and prone to errors. Need a more streamlined approach for your PWA development? Google’s Workbox library set offers a practical solution.
Widely adopted by developers, Workbox provides higher-level abstractions over the standard Service Worker API, significantly simplifying common tasks. Its modules help manage:
- Precaching: Automatically caches essential assets (like your App Shell) during the service worker installation, ensuring they load instantly.
- Runtime Caching: Offers multiple (at least five) built-in strategies (e.g., Cache First, Network First, Stale-While-Revalidate) to flexibly handle how dynamic content and API responses are cached during use.
- Routing: Allows you to easily define rules matching URL patterns or request types to specific caching strategies.
- Background Sync: Includes a dedicated module (workbox-background-sync) that simplifies queuing failed network requests made offline and retrying them automatically upon reconnection.
- Build Tool Integration: Provides plugins for popular tools like Webpack and Rollup, plus a command-line interface (CLI), enabling automatic service worker generation based on your project’s assets and configuration.
By handling common patterns and lifecycle management, Workbox helps US developers implement robust offline capabilities and other advanced PWA features more efficiently, requiring less boilerplate code compared to using the raw Service Worker APIs directly.
Transpilers: Babel
How can you leverage the power and cleaner syntax of modern JavaScript (ES6/ES2015 and newer) in your PWA while still ensuring it runs correctly on the wide range of browsers used across the US, including those that haven’t fully caught up? Transpilers are the key, and Babel is the most widely adopted solution.
Babel functions as a JavaScript compiler. It takes your modern code, written with features like arrow functions, classes, or async/await, and transforms it into an equivalent version using older syntax (typically ES5). This older ES5 standard enjoys nearly universal support across browsers, providing a reliable compatibility baseline.
Integration is typically handled within your project’s build process, often via plugins for tools like Webpack or Rollup. You configure Babel (using a .babelrc file or similar settings) to specify which browser versions you need to support, ensuring your PWA’s JavaScript works correctly across your target audience. This allows developers to write up-to-date code without sacrificing reach.
Polyfills:
So, transpilers like Babel handle differences in JavaScript syntax, but what if a browser completely lacks a modern feature or API your PWA needs? If some users in the US market rely on browsers without built-in Promise support or the Workspace API, how do you bridge that gap? That’s the role of Polyfills.
A polyfill is a piece of code (typically JavaScript) that simulates the behavior of a native web API or method when it’s missing in the user’s browser. They enable you to code against modern standards, but unlike transpiled syntax which often has minimal overhead, polyfills add code to provide missing functionality.
Common examples where polyfills might be considered include:
- ES6+ Features: Functions like Object.assign(), Array.from(), or objects like Promise and Symbol.
- Fetch API: While native support for Workspace() is now widespread (reducing the need for its polyfill compared to a few years ago), older environments might still require it.
- Other Web APIs: Depending on PWA features, you might need polyfills for APIs like Intersection Observer or URL.
The trade-off is performance: polyfills increase JavaScript bundle size (potentially adding several kilobytes or more per feature) and can introduce overhead. Use them strategically:
- Load Conditionally: Employ feature detection to load a polyfill only if the native API is absent.
- Target Precisely: Utilize services like Polyfill.io or configure tools like Babel’s @babel/preset-env to bundle only the specific polyfills needed for your defined target browsers, avoiding unnecessary bloat.
PWA Scaffolding/Auditing Tools
Whether you’re starting a new PWA project or need to ensure an existing web application meets the necessary criteria, several tools can accelerate development and validation for US-focused applications.
Consider incorporating these into your workflow:
- PWA Builder: This Microsoft toolset assists across the PWA lifecycle. It helps initiate projects, validate existing sites, generate essential components like Web App Manifests and basic service workers, create necessary icon sizes, and even package PWAs for distribution in app stores like the Microsoft Store and Google Play.
- Lighthouse: Integrated directly into Chrome DevTools (and available via CLI/Node), Google’s Lighthouse is an indispensable auditing tool. It evaluates your PWA against five core areas: Performance (including Core Web Vitals), Accessibility, Best Practices, SEO, and specific PWA installability requirements. Lighthouse provides scores (0-100) and actionable advice for improvement in each category.
- Framework-Specific Tooling: If you’re using popular front-end frameworks like React, Angular, Vue, or Next.js, explore their dedicated PWA modules or plugins. These often streamline setup by integrating manifest generation and service worker configuration (frequently using Workbox) directly into the framework’s build process.
Leveraging these tools can significantly simplify setup, identify areas for improvement, and help ensure your PWA adheres to current best practices.
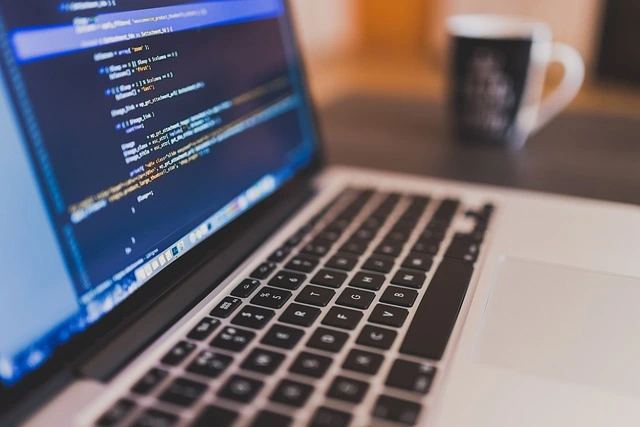
Practical Guidelines and Workarounds
BSuccessfully launching a Progressive Web App that performs well across the diverse US browser and device landscape involves applying best practices strategically. Here’s a consolidation of key recommendations:
Foundational Strategy: Progressive Enhancement & Feature Detection
- Build Layer by Layer: Start your PWA with a core of semantic HTML delivering essential content and function universally. This ensures baseline usability on virtually any browser.
- Enhance Progressively: Layer CSS for presentation, then JavaScript for richer interactivity. Add advanced PWA features (offline access, push notifications) as further enhancements.
- Check Before Using: Always employ robust feature detection to verify browser support before attempting to use a specific API or CSS feature. This prevents errors and maximizes reach.
Performance is Non-Negotiable
- Aim for Speed: Treat performance as a primary feature. Target meeting Core Web Vitals thresholds (e.g., LCP under 2.5s) for a good user experience.
- Optimize Everything: Aggressively minify code (JS, CSS) and compress images using modern formats (WebP, AVIF) and responsive techniques (srcset).
- Load Smart: Use code splitting and lazy loading to minimize initial download sizes.
- Cache Strategically: Leverage service workers (via Workbox) for caching. Implement the App Shell pattern for instant UI loading and use appropriate runtime caching strategies (e.g., Stale-While-Revalidate) for dynamic content.
- Audit Continuously: Regularly test performance with Lighthouse and browser DevTools, particularly simulating varied US network conditions (like slower mobile connections).
Designing Robust Offline Experiences
- Minimum Viable Offline: Replace the generic browser offline error with a custom, user-friendly offline page via a service worker.
- Enable Core Functionality: Use a service worker (Workbox recommended) to cache the App Shell, critical assets (JS/CSS), and essential data, aiming for near-100% uptime for key features offline.
- Store Offline Data: Utilize IndexedDB for storing significant amounts of user-generated data or application state needed offline.
- Communicate Status: Clearly indicate to the user whether the application is currently online or offline.
- Handle Offline Submissions: Use the Background Sync API for deferred data syncing (like form posts) primarily on supported Chromium browsers. Crucially, always implement a fallback: store pending data locally (e.g., IndexedDB) and sync from the main thread when navigator.onLine shows connectivity is back, due to Background Sync’s limited support elsewhere.
Strategizing for iOS Limitations
- Acknowledge Differences: Recognize upfront that PWAs on Safari/iOS have limitations compared to other platforms. This is critical given iOS holds a significant mobile browser share (around 55-60%) in the US.
- Guide Installation: Provide clear, simple visual instructions for the manual “Add to Home Screen” process via the Share menu.
- Manage Push Expectations: Implement Push API for iOS 16.4+ (only after A2HS), but understand its delivery might be less reliable than on other platforms; consider alternatives if guaranteed delivery is vital.
- Avoid Unsupported APIs: Do not rely on Background Sync or certain other device APIs. Use feature detection diligently.
- Test Extensively: Thoroughly test functionality, layout, and storage behavior across various iOS versions and devices.
- Make the Call: Based on testing and requirements, decide if the PWA feature set on iOS is sufficient, or if alternatives (native wrappers, full native) are needed for that segment.
Leveraging the Right Tools
- Simplify Service Workers: Use Workbox for efficient caching, background sync, and lifecycle management.
- Ensure Compatibility: Employ Babel for transpiling modern JavaScript. Use Polyfills selectively only where needed, monitoring bundle size impact.
- Audit & Debug: Regularly use Lighthouse for PWA compliance checks and browser DevTools for debugging and performance analysis.
- Broaden Test Coverage: Consider cloud-based testing platforms (like BrowserStack, Sauce Labs) to efficiently test across a wide range of browsers, devices, and OS versions prevalent in the US.
Comprehensive and Continuous Testing
- Test Early, Test Often: Integrate testing throughout the development lifecycle.
- Use Multiple Layers: Combine manual testing (for UX/visuals on key devices), automated functional/regression tests (Selenium, Playwright), and real-device testing.
- Focus on PWA Features: Specifically test offline behavior (simulate via DevTools), service worker activation/updates, caching logic, the Add to Home Screen flow, push notifications, and background sync fallbacks.
- Prioritize Targets: Base your primary testing efforts on usage statistics reflecting your US target audience (e.g., aim to cover browsers representing >95% of users).
Managing Service Worker Updates Smoothly
- Understand the Lifecycle: Learn how service workers update, including the default “waiting” state where a new worker won’t activate until all old tabs/windows are closed.
- Decide on skipWaiting(): Carefully consider using self.skipWaiting() to force immediate activation, understanding it might cause temporary inconsistencies if not handled well.
- Prevent Cache Conflicts: Use cache-busting techniques (like hashing filenames) for static assets. Implement logic in the activate event of your new service worker to clean up old, unused caches, preventing storage bloat.
- Inform the User: Consider adding a UI element (like a banner or button) to notify users when a new PWA version is ready, allowing them to refresh and activate it conveniently.
Conclusion
Progressive Web Apps offer significant advantages, delivering installable, offline-capable experiences using web technology. However, building reliable PWAs requires navigating cross-browser inconsistencies, particularly the limitations on iOS Safari which impacts a large segment of the US mobile market.
Success hinges on a strategic approach: prioritize Progressive Enhancement and Feature Detection, relentlessly optimize for performance (targeting Core Web Vitals), test across diverse US user environments, and leverage tools like Workbox and Lighthouse effectively.
While challenges exist, robust PWAs are achievable. The platform continues to evolve (e.g., Project Fugu adding capabilities), making adaptable practices key.
Optimize Your PWA Strategy
Ready to build a more effective PWA for the US market? Let’s discuss how our expertise can help.
Schedule your complimentary 2-hour consultation session today.