Tangled in complex data hierarchies like nested categories? Test-Driven Development (TDD) offers a lifeline, reportedly slashing bug density by 40-80%. This post explores applying TDD in Ember.js—leveraging its robust testing tools—to master tricky reflexive relationships (models relating to themselves). We’ll cover unit to acceptance testing for building robust, maintainable web applications.
Test-Driven Development (TDD) flips traditional coding on its head: you write tests before your actual code. This iterative practice drives simpler designs and ensures code correctness from the start.
Table of Contents
Understanding Test-Driven Development (TDD)
The Core Cycle: Red-Green-Refactor
TDD revolves around this rapid, iterative loop:
- Red: Write a test for a new function. It fails initially because the code doesn’t exist.
- Green: Write the minimum code needed to make the test pass.
- Refactor: Improve the code’s structure and clarity, with tests ensuring no breakage.
Key Principles & Significant Payoffs
TDD builds on principles like ‘test first’ and ‘small increments.’ This focus yields substantial benefits:
- Early Bug Detection: Catches issues pre-code or early in development. Addressing bugs found late in a project can cost up to 30 times more than if caught during design or early coding – TDD significantly mitigates this.
- Improved Design: Leads to modular, maintainable, and simpler code, avoiding over-engineering.
- Living Documentation: Tests act as up-to-date, executable specifications.
- Confident Refactoring: A comprehensive test suite allows for fearless code improvements.
How TDD Differs from Traditional Testing
- Timing: TDD tests precede code; traditional tests follow development.
- Focus: TDD emphasizes unit tests during development; traditional testing often focuses on broader tests later.
- Process: TDD is iterative with constant feedback; traditional can be more linear.
- Debugging: TDD simplifies debugging by isolating errors to recent, small changes.
TDD integrates testing as a core design activity, not just a final quality check.
Understanding Reflexive Relationships and Ember Data
Reflexive relationships—where a data model links to itself (e.g., categories with sub-categories, threaded comments)—are common for building hierarchical or graph-like structures. Ember.js, via Ember Data, offers robust tools for managing these complex structures efficiently.
Ember Data: Key Concepts for Managing Relationships
Ember Data simplifies data persistence and relationships:
- Models: Define your application data structure, including attributes and connections.
- Store: Acts as a central cache for your records, ensuring data consistency across your application. Ember Data’s conventions can reduce data management boilerplate and, with disciplined use, contribute to fewer data-related consistency issues.
- Relationships: Use decorators like @hasMany(‘self-model-name’) and @belongsTo(‘self-model-name’) to define how model instances connect to other instances of the same type.
Implementing Reflexive Links in Ember Data Models
A model can establish relationships with other instances of itself. Consider a folder model:
JavaScript
// app/models/folder.js
import Model, { attr, belongsTo, hasMany } from ‘@ember-data/model’;
export default class FolderModel extends Model {
@attr(‘string’) name;
@hasMany(‘folder’, { inverse: ‘parent’, async: true }) children;
@belongsTo(‘folder’, { inverse: ‘children’, async: true }) parent;
}
Key options when defining these self-referential links:
- inverse Option: Essential for reflexive relationships. It explicitly tells Ember Data how the two sides connect (e.g., a folder’s children property is the inverse of another folder’s parent property). This is crucial for Ember Data to automatically maintain data consistency when relationships are updated.
- async Loading: Determines how related data is fetched.
- async: true (default for @hasMany): Related records are loaded only when the relationship property is accessed, returning a promise. This improves initial performance by deferring data fetching.
- async: false: Ember Data expects related records to be already loaded (e.g., sideloaded). Accessing the property returns records directly, not a promise.
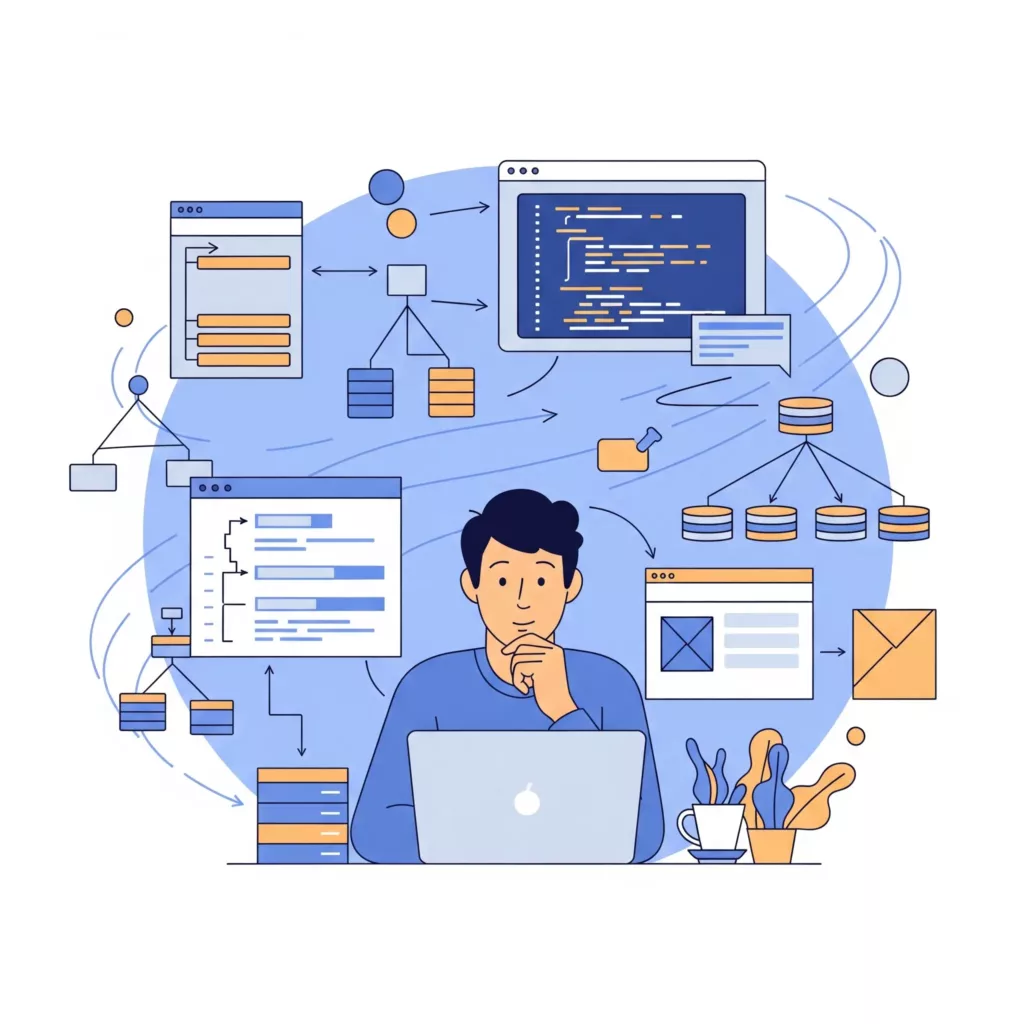
TDD Workflow for Reflexive Relationships in Ember.js
Applying Test-Driven Development (TDD) to Ember.js features with reflexive relationships (where a model relates to itself, like categories with sub-categories) ensures robust, maintainable code. Ember’s testing tools, particularly with QUnit, support this across different testing levels. Effective TDD here can catch data integrity issues early, preventing bugs that are often significantly more costly to fix post-deployment.
Ember.js Test Types for Reflexive Relationships
Ember provides a clear testing pyramid. For reflexive relationships, consider:
Test Type | Scope & Purpose | Speed | Key Ember Helpers | Use for Reflexive Relationships |
Unit | Isolated logic in models, services. Verifies specific functions. | Fastest | setupTest, this.owner.lookup(), store methods | Testing model relationship definitions (@hasMany, @belongsTo, inverse), custom model logic. |
Rendering | Component appearance, interactions, and lifecycle hooks. Uses rendering engine. | Medium | setupRenderingTest, render, click, hbs | Testing components displaying hierarchies (e.g., trees), node interactions (expand/collapse). |
Application | End-to-end user flows through the entire app. Simulates full user interaction. | Slowest | setupApplicationTest, visit, click, fillIn | Testing user scenarios like creating/moving/deleting items in a hierarchy across views. |
TDD Workflow Highlights
The core TDD cycle (Red-Green-Refactor) applies at each level:
- Unit Testing Models (e.g., a category model):
- Red (Write Failing Test): Assert that the category model correctly defines its parent (@belongsTo) and children (@hasMany) relationships, including the crucial inverse option.
- Green (Make Test Pass): Implement these relationships in your category.js model file.
JavaScript
// app/models/category.js - import Model, { attr, belongsTo, hasMany } from ‘@ember-data/model’;
- export default class CategoryModel extends Model {
- @attr(‘string’) name;
- @belongsTo(‘category’, { async: true, inverse: ‘children’ }) parent;
- @hasMany(‘category’, { async: true, inverse: ‘parent’ }) children;
- }
- Refactor: Clean up.
- Why it Matters: Ensures foundational data integrity. Incorrect inverse options are a common source of hard-to-debug state inconsistencies in Ember Data. TDD catches these definitions errors immediately. Also, test any custom model logic for manipulating these hierarchies (e.g., an addChild method), paying attention to how async relationships (returning promises) are handled.
- Rendering Hierarchical Components (e.g., a category-item component):
- TDD Cycle: Start by testing the display of a single item. Then, write a failing test for rendering child items recursively if the category has children. Implement the recursive logic in your Handlebars template ({{#each @category.children as |childCategory|}} <CategoryItem @category={{childCategory}} /> {{/each}}). Test interactions like expanding/collapsing nodes.
- Why it Matters: Verifies the UI correctly reflects the data structure. TDD encourages well-defined component interfaces (arguments and actions) necessary for recursion. Ember CLI Mirage is invaluable here for providing consistent hierarchical data to your rendering tests.
- Application Testing User Scenarios (e.g., managing categories):
- TDD Cycle: For a feature like “creating a sub-category,” write a test that simulates user actions: visit(‘/categories/new’), fillIn a name, select a parent, click save. Assert the new sub-category appears correctly in the hierarchy and the URL is correct. Implement the route, template, form, and data-saving logic.
- Why it Matters: Confirms all parts of your application (routes, models, components, services) work together for complex user flows involving reflexive data. These tests often highlight challenges in data loading strategies for nested data and heavily rely on robust API mocking with tools like Ember CLI Mirage.
By applying TDD systematically, you build confidence in your Ember.js application’s ability to handle complex reflexive relationships, leading to higher quality and more maintainable code.
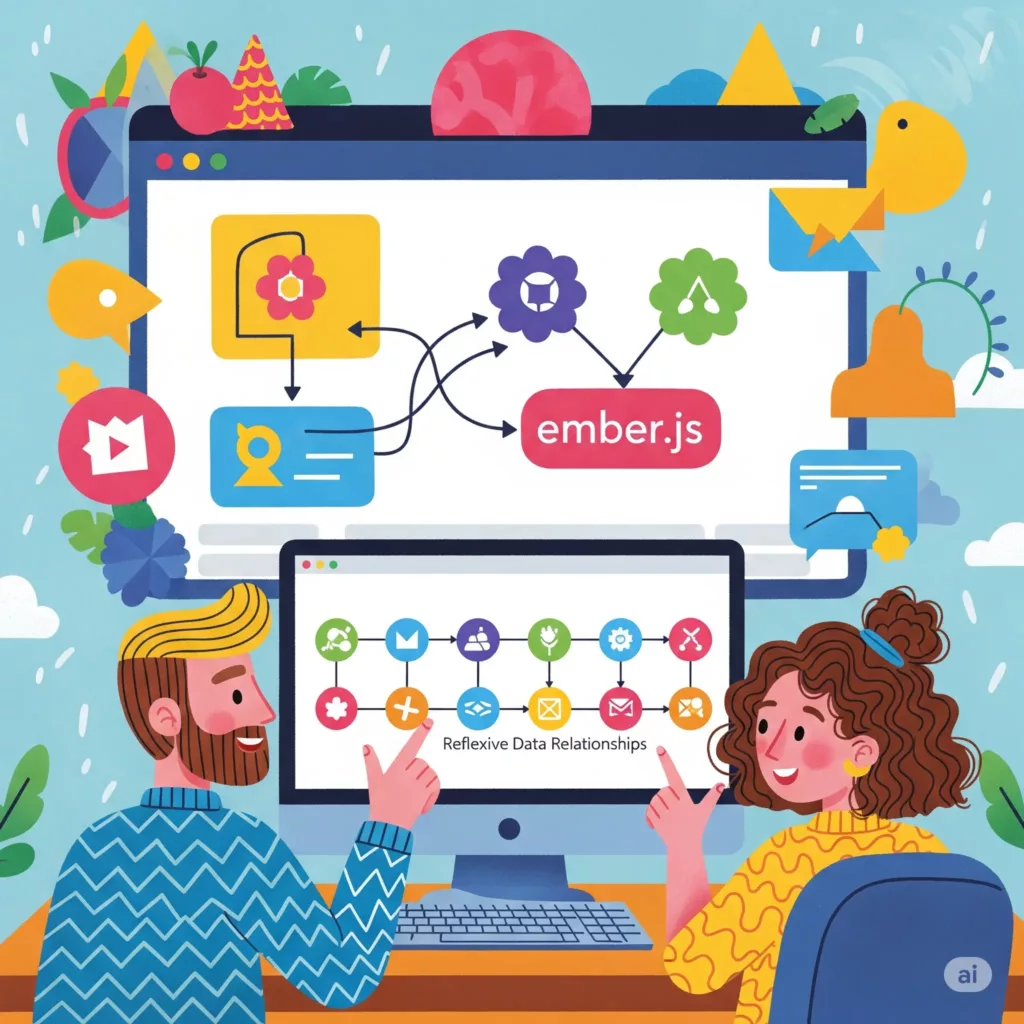
Advanced Considerations and Best Practices
Developing robust Ember.js applications with reflexive relationships (e.g., nested categories, organizational charts) requires mastering several advanced concepts beyond basic setup. Here’s what to focus on for data consistency, performance, and reliability.
1. Ensuring Data Consistency in Updates
When modifying reflexive relationships (like changing an item’s parent), data integrity is key.
- Master inverse Relationships: Correctly defined inverse options in your Ember Data models are crucial. They allow Ember Data to automatically manage client-side consistency across both sides of the relationship.
- Server Synchronization: Ensure your server API responses accurately reflect all relationship changes to keep the Ember Data store in sync and prevent stale data.
- TDD for Updates: Write tests that modify relationships, save records, and then assert that all affected parties (the item, its old parent, its new parent) are correctly updated in the store.
2. Smart Serialization Strategies
Serializing reflexive relationships for APIs can lead to circular references or excessive payload sizes.
- Adopt JSON:API: This specification, well-supported by Ember Data’s JSONAPISerializer, handles relationships using links or sideloaded included data, mitigating circular reference issues and helping manage payload size. Its adoption is widespread for building robust APIs.
- Customize Carefully: If not using JSON:API, customize serializers (e.g., with EmbeddedRecordsMixin) to control embedding depth and prevent cycles, often by sending relationship IDs beyond a certain nesting level.
- API Controls: Leverage API features like include parameters for controlled data fetching.
3. Optimizing Performance with Large Hierarchies
Deeply nested or wide hierarchies can strain performance.
- Combat N+1 Queries: Unoptimized N+1 queries (accessing an async relationship for each item in a loop) can add hundreds of milliseconds, or even seconds, to load times. Use Ember Data’s include option for eager loading or explicit store.query() calls with pagination for large hasMany relationships.
- Efficient Rendering: For extensive lists or trees, employ UI virtualization (e.g., with ember-collection). These techniques can make rendering lists with thousands of items feel instantaneous, often reducing initial render times by over 90%.
- Data Representation: For read-only deep hierarchies, consider using plain JavaScript objects via DS.attr() instead of full Ember Data models for every level to reduce overhead.
4. Handling Cascading Deletes Safely
Define clear behavior when a record in a hierarchy is deleted (e.g., cascade delete to children, nullify parent links, or prevent deletion).
- Server-Side Priority: Robust cascading deletes are often best handled server-side via database constraints (e.g., ON DELETE CASCADE) for transactional safety.
- Client-Side Addons: Tools like ember-data-cascade-delete can manage client-side store cleanup but require careful configuration to avoid issues like infinite loops in reflexive relationships.
- TDD for Deletes: Test various scenarios (deleting leaf nodes, nodes with children, nodes with deep hierarchies) to verify the implemented logic.
5. Avoiding Common Pitfalls & Effective Debugging
- Key Pitfalls: Misconfigured inverse properties, mishandling async relationship promises, complex Mirage factory setup for hierarchies, and outdated dependencies are common pain points.
- Debugging Toolkit:
- Ember Inspector: Essential for inspecting the store, relationships, and component states.
- await pauseTest();: In tests, this helper halts execution for live debugging in the browser.
- Systematic TDD: Helps pinpoint bugs to recent, small code changes.
Mastering these areas will enable you to build sophisticated, performant, and reliable Ember.js applications featuring complex, self-referential data structures.
Conclusion
Mastering reflexive relationships in Ember.js using Test-Driven Development isn’t just about cleaner code; it’s a strategic advantage. By consistently applying TDD, developers can slash bug rates by a reported 40-80%, leading to highly robust and maintainable web applications. This disciplined approach transforms complex hierarchical data management from a challenge into a strength for your Ember projects.
For more deep dives and practical insights into building cutting-edge web applications, visit our blog.