Android powers over 3 billion devices globally in 2025. What’s Java’s core Object-Oriented Programming (OOP) secret to this scale? Java’s OOP principles were pivotal in shaping Android’s architecture, enabling robust, scalable apps. Core concepts like encapsulation and inheritance, though often now applied via Kotlin, remain vital for developers. Understanding these foundational Java OOP tenets is crucial for effective Android development in 2025. This guide explores their lasting impact and practical application in building maintainable mobile solutions.
Table of Contents
Foundations of Java Object-Oriented Programming
A clear understanding of Java’s core Object-Oriented Programming (OOP) tenets is essential before exploring their application within the Android framework. These principles are foundational for designing modular, reusable, and maintainable software, critical in the complex world of mobile app development in 2025.
The following table provides a concise overview of these core principles and their relevance in Android:
Principle | Brief Definition | Example Android Application |
Class | A blueprint or template for creating objects, defining properties (attributes) and methods (behaviors). | The android.app.Activity class serves as a blueprint for individual app screens. |
Object | An instance of a class, representing an entity with state and behavior. | An instance of MainActivity (a custom class extending Activity) is an object representing a specific app’s main screen. |
Encapsulation | Bundling data and methods within a class, restricting direct access to internal components. | An Activity object manages its internal lifecycle state, exposing controlled methods like onCreate() for interaction. |
Inheritance | A mechanism where a new class (subclass) acquires properties and behaviors from an existing class (superclass). | A custom MyCustomButton class extending android.widget.Button to add new features. |
Polymorphism | The ability of an object to take on many forms; a single interface/method can be used for various actions. | Different View objects (e.g., TextView, ImageView) all responding uniquely to the onDraw() method. |
Abstraction | Hiding complex implementation details, showing only essential features or functionalities of an object. | Calling setContentView(R.layout.main_activity) without needing to know the details of XML parsing and view inflation. |
Classes and Objects: Defining Blueprints and Instances
A class is a blueprint for creating objects, defining common properties (attributes) and behaviors (methods). For example:
Java
public class Dog {
// Attributes
String breed;
int age;
// Methods
void bark() { System.out.println(“Woof!”); }
void fetch(String item) { /* … */ }
}
An object is a concrete instance of a class, residing in memory. Creating an object (e.g., Dog myDog1 = new Dog();) allocates memory. myDog1 and myDog2 would be distinct Dog objects.
Android applications are collections of interacting objects. Core components like Activity, Service, Fragment, and View are all classes. Developers build apps by instantiating these or their own custom subclasses. Every screen a user interacts with is typically an object of an Activity subclass. This deep integration makes understanding classes and objects indispensable for Android development.
Encapsulation: Protecting Data and Bundling Behavior
Encapsulation bundles an object’s data and its operating methods into a single unit (the class), often restricting direct access to internal data to protect its state—a principle known as data hiding. This ensures data is modified only through well-defined interfaces.
In Java, this is achieved using access modifiers (e.g., private). Public “getter” and “setter” methods provide controlled access. Consider a BankAccount:
Java
public class BankAccount {
private double balance; // Encapsulated
public double getBalance() { return this.balance; }
public void deposit(double amount) { if (amount > 0) this.balance += amount; }
public boolean withdraw(double amount) { /* … validation … */ }
}
Here, balance is private, accessed and modified only via methods that can include validation logic, enhancing data integrity.
In Android, encapsulation is foundational for component independence and security (e.g., the application sandbox model). An Activity’s internal state is managed internally, with interactions via well-defined lifecycle callbacks, preventing arbitrary cross-component interference and bolstering system stability.
Inheritance: Code Reusability and Hierarchical Classification
Inheritance allows a new class (subclass) to acquire attributes and behaviors from an existing class (superclass), promoting code reusability and creating an “is-a” relationship (e.g., a Car is a Vehicle). Java uses the extends keyword.
Java
class Vehicle {
public void startEngine() { /* … */ }
}
class Car extends Vehicle {
@Override // Method Overriding
public void startEngine() { System.out.println(“Car engine started.”); }
public void openSunroof() { /* … */ }
}
The Car class inherits from Vehicle and can add or override behaviors.
Inheritance is the backbone of Android’s UI framework. UI elements like TextView and Button all inherit from a base View class, reusing common functionalities. Developers extend core components like Activity or Service to implement app-specific logic. This extensibility accelerates development and ensures a consistent framework across the vast Android ecosystem, supporting millions of apps.
Polymorphism: Achieving Flexibility through “Many Forms”
Polymorphism allows a single action to be performed in different ways depending on the object.
Compile-time Polymorphism (Method Overloading): Multiple methods in a class share the same name but have different parameter lists. The compiler chooses the correct one.
- Java
class Display {
public void show(String message) { /* … */ }
public void show(String message, int times) { /* … */ }
}
Runtime Polymorphism (Method Overriding):
A subclass provides a specific implementation for a method defined in its superclass. The choice occurs at runtime based on the object’s actual type.
- Java
abstract class Shape { abstract void draw(); }
class Circle extends Shape { @Override void draw() { /* Draw Circle */ } }
class Square extends Shape { @Override void draw() { /* Draw Square */ } }
// Usage: Shape s = new Circle(); s.draw(); // Calls Circle’s draw
Polymorphism is key to Android’s generic UI handling. Common methods like onDraw() in the base View class are overridden by subclasses (Button, TextView) to provide unique rendering. Similarly, Android’s event listener mechanism (e.g., View.OnClickListener) uses polymorphism via interfaces, allowing different UI elements to have unique click actions, making the framework flexible and extensible.
Abstraction: Simplifying Complexity and Focusing on Essentials
Abstraction hides complex implementation details, exposing only essential functionalities. Users interact with simplified interfaces without needing to understand internal workings (e.g., driving a car without knowing engine mechanics).
In Java, abstraction is achieved via:
Abstract Classes: Cannot be instantiated; may contain abstract methods (no implementation) that subclasses must implement.
- Java
abstract class Animal {
public abstract void makeSound(); // Abstract method
public void sleep() { /* … */ } // Concrete method
}
class Lion extends Animal { @Override public void makeSound() { System.out.println(“Roar!”); } }
Interfaces: Blueprints containing (traditionally) only abstract method declarations. Classes implement interfaces, providing concrete method implementations.
- Java
interface Drivable { void drive(); void stop(); }
class MyCar implements Drivable { /* … provides drive() and stop() … */ }
Abstraction is pivotal for the Android SDK’s usability and device independence. Developers use high-level APIs (e.g., CameraManager, SensorManager) without needing device-specific code. UI rendering is also abstracted; developers define UIs (XML or Jetpack Compose), and the system handles complex drawing and adaptation. This enhances productivity and allows the platform to evolve without breaking existing apps, crucial for an ecosystem with billions of devices.
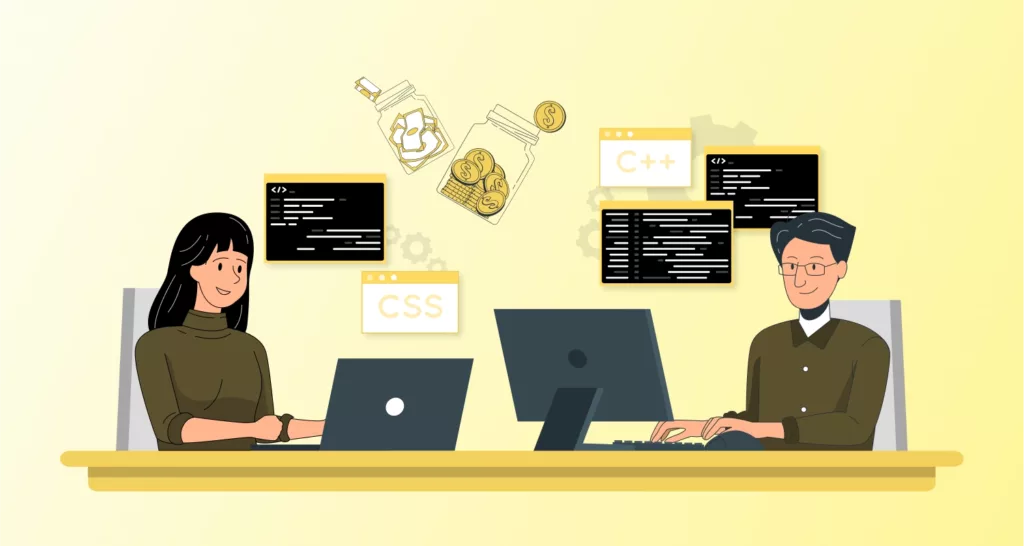
Java OOP Principles in Android Application Development
ava’s foundational Object-Oriented Programming (OOP) principles—Inheritance, Encapsulation, Polymorphism, and Abstraction—are pervasively applied throughout the Android application development lifecycle, shaping how developers build components and interact with the Android framework, which underpins an ecosystem supporting billions of devices globally.
Inheritance in Android: Extending Core Classes
Inheritance is a cornerstone, enabling code reuse and specialization by building upon the Android framework’s base classes.
- Extending Activity: Developers create app screens by extending android.app.Activity or, more commonly for modern UI features and backward compatibility, androidx.appcompat.app.AppCompatActivity. Custom activities inherit lifecycle management (e.g., onCreate(), onPause()) and UI capabilities. Every screen across millions of Android apps is typically an instance of such a subclass. Example: public class MainActivity extends AppCompatActivity { /* … */ }
- Extending View / ViewGroup: For custom UI components with unique appearances or behaviors, developers extend base View or ViewGroup classes (e.g., Button, LinearLayout), often overriding methods like onDraw(), onMeasure(), and onTouchEvent().
- Extending Fragment: Reusable UI portions within an Activity are created by extending androidx.fragment.app.Fragment or specialized subclasses like DialogFragment.
- Extending Other Core Components: Similar patterns apply to Service (background tasks), BroadcastReceiver (system broadcasts), and ContentProvider (data sharing).
The Android SDK provides comprehensive base classes, defining contracts and core functionalities. By requiring developers to extend these, the framework ensures adherence to established patterns (like lifecycle methods), allowing developers to leverage pre-built functionality while focusing on application-specific logic. This balances a structured framework with high customization.
Encapsulation in Managing Android Components’ State and Interactions
Encapsulation is critical for managing Android component complexity by bundling data and behavior and controlling internal state access.
- Activity Encapsulation: An Activity manages its own lifecycle (created, started, resumed, etc.), internal state (user data, scroll positions), and UI interactions. Developers interact via well-defined lifecycle callbacks (e.g., onCreate(), onSaveInstanceState()), hiding internal transition mechanics.
- View Encapsulation: Each View object (e.g., TextView, Button) encapsulates properties like visibility and text content, accessed and modified via public methods (TextView.setText(), View.setVisibility()).
- Fragment Encapsulation: Fragments are modular, managing their own layout, lifecycle, and input events. Communication often occurs indirectly via shared ViewModel objects or the FragmentResult API to maintain encapsulation.
- Data Models and ViewModel: Custom Java/Kotlin classes encapsulate application data. Modern Android architecture uses ViewModel to store and manage UI-related data in a lifecycle-conscious way, separating it from UI controllers and protecting it during configuration changes.
Encapsulation enables Android’s effective lifecycle and resource management. The OS can destroy and recreate components like Activities (e.g., during screen rotation) trusting them to save/restore essential data via defined interfaces (like onSaveInstanceState()). This separation of concerns contributes significantly to app stability.
Polymorphism in Event Handling and Method Overriding
Polymorphism allows objects of different types to respond uniquely to the same message or method call, providing flexibility.
- Method Overriding in Lifecycle Callbacks: Android components like Activity and Fragment have base lifecycle methods (e.g., onCreate(), onStart()). Developers override these in subclasses to inject specific behaviors. The framework calls the appropriate overridden method at runtime.
- View.onDraw() and UI Rendering: The onDraw(Canvas canvas) method in the base View class is overridden by every View subclass (TextView, ImageView, custom views) to implement specific rendering logic. The system calls onDraw() on each view, and each polymorphically draws itself.
Event Listeners (Callback Mechanism): Android’s event system relies on polymorphism via interfaces like View.OnClickListener. Different UI elements can have distinct listener objects implementing unique actions for the same event type (e.g., a click). Example:
Java
saveButton.setOnClickListener(v -> { /* Logic to save data */ });
cancelButton.setOnClickListener(v -> { /* Logic to cancel */ });
- Both buttons respond to onClick(), but with different actions due to different listener implementations.
- Handling Different Intents: An Activity can polymorphically adapt its behavior based on the action or data within the Intent that started it or is delivered via onNewIntent().
Polymorphism, especially via interfaces in event listeners, creates a decoupled and extensible event model, allowing a clean separation between UI presentation and business logic.
Abstraction in the Android SDK: Simplifying Complex Operations
Abstraction simplifies development by hiding complex underlying mechanisms and exposing only essential functionalities. The Android SDK is rich with abstractions:
- UI Rendering Abstraction: Developers use XML layouts or Jetpack Compose to define UIs. The framework abstracts the complexity of parsing, drawing, handling screen densities, and hardware acceleration (e.g., via Activity.setContentView()).
- Window Management Abstraction: The Activity class abstracts Android’s underlying window management system.
- Hardware Access Abstraction: High-level APIs (CameraManager, LocationManager, SensorManager) allow interaction with device hardware without needing device-specific driver code, crucial for the diverse Android hardware ecosystem.
- Resource Management Abstraction: Android’s resource system (e.g., R.string.app_name, R.drawable.icon) abstracts asset management, automatically loading appropriate resources based on device configuration.
- Concurrency and Background Task Abstraction: Modern solutions like ViewModels with Kotlin Coroutines or LiveData offer high-level abstractions for managing asynchronous tasks and UI updates safely.
These abstraction layers enhance developer productivity and platform stability, allowing developers to focus on application logic rather than system internals. This enables Google to evolve the underlying platform without necessarily breaking existing applications, promoting forward compatibility across the vast Android device landscape.
Advantages of Employing OOP in Android Development
Adopting Java’s Object-Oriented Programming (OOP) principles in Android development offers numerous advantages, contributing to well-structured, efficient, and maintainable applications—essential in the competitive 2025 app landscape.
Key Advantages of OOP in Android Development
- Significant Code Reusability OOP tenets like inheritance and composition enable developers to reuse existing, tested code, drastically reducing redundancy and accelerating development time. For instance, a base Activity class with common features (toolbar setup, navigation) can be inherited by specific app activities, saving considerable repetitive coding. Well-designed custom UI views can also be reused across multiple screens or projects. This efficient reuse can often lead to a 15-25% reduction in initial development efforts for common functionalities.
- Enhanced Modularity OOP encourages breaking down complex applications into smaller, self-contained modules (typically classes or packages). Each module handles specific functionality (e.g., Product management, User authentication, Order processing in an e-commerce app). This clear separation simplifies development and understanding.
- Improved Maintainability The modularity and encapsulation fostered by OOP result in applications that are easier to maintain and update. Changes or bug fixes can often be isolated to specific modules, minimizing the risk of unintended side effects. Industry observations suggest that modular systems, a key OOP benefit, can correlate with up to a 20-30% reduction in bug-fixing time and lower long-term maintenance costs.
- Greater Scalability OOP-designed applications are generally more scalable, allowing them to evolve gracefully to accommodate new features without extensive rewrites. Well-defined interfaces between objects make it easier to integrate new modules or extend existing ones (e.g., adding a new shipping option to an e-commerce app).
- Increased Readability and Simplified Debugging Well-structured OOP code, with clear responsibilities for each class, tends to be more readable and easier to understand. This, in turn, simplifies debugging, as issues can often be traced more quickly to specific objects or methods.
- Flexibility and Robustness in Design Polymorphism allows for flexible designs that adapt to changing requirements or diverse data types through common interfaces. Encapsulation protects an object’s internal state and controls data access, contributing to overall application robustness by ensuring predictable behavior.
- Natural Alignment with Android’s Architecture Android’s fundamental architecture is built around distinct, interacting components (Activities, Services, etc.). Java’s OOP nature aligns perfectly, providing a natural way to define and manage these components as objects.
- Facilitation of Proven Design Patterns OOP principles underpin many established software design patterns (e.g., Singleton for a database helper, Factory for object creation, Observer for event-driven updates like with LiveData). These patterns help solve recurring problems and create more robust, flexible applications.
Impact on Large-Scale Projects and Testability
These OOP benefits are particularly pronounced in large-scale Android projects. Modularity allows concurrent development with reduced interference. Code reusability ensures consistency and saves effort. Crucially, strong OOP design enhances maintainability and scalability, vital for an app’s long-term lifecycle involving bug fixes, OS updates, and new features.
Furthermore, good OOP practice significantly improves codebase testability. Encapsulated classes with clear interfaces are easier to unit test. Techniques like Dependency Injection, often used with OOP, further enhance testability by allowing dependency mocking. This focus on testable, modular components contributes to higher software quality, fewer regressions, and a more confident development process, making robust OOP a necessity for sustainable, enterprise-level Android applications.
Conclusion
Java’s Object-Oriented Programming (OOP) principles formed the bedrock of Android’s architecture, underpinning the success of millions of apps globally. In 2025, understanding how encapsulation, inheritance, polymorphism, and abstraction translate into maintainable, scalable, and efficient Android applications remains crucial for developers. Mastering these OOP concepts is key to moving from simply coding to truly architecting superior, high-quality mobile experiences that stand the test of time.
Ready to build an Android app with a strong, future-proof OOP foundation? Partner with us; our deep Java expertise ensures your application is not just functional, but exceptionally well-architected for lasting success.