This article delves into the development of Ruby on Rails applications specifically designed to function as an API for a React frontend. This architectural pattern combines the strengths of both frameworks, capitalizing on Rails’ robust backend capabilities and database management, and React’s proficiency in crafting dynamic and interactive user interfaces.
Before we dive into the specifics, let’s first understand what an API is and how it works. An API, or Application Programming Interface, allows different applications to communicate and exchange data. This communication typically follows a request-response cycle: a user interacts with an application, triggering a request for data; this request is sent to the API, which retrieves the data and sends it back to the user.
Table of Contents
Why Use Ruby on Rails for API Development?
Ruby on Rails has emerged as a popular framework for building APIs due to its numerous advantages:
- Rapid Development: Rails adheres to the “Convention over Configuration” principle and the “Don’t Repeat Yourself” (DRY) principle. By providing sensible defaults and encouraging concise, reusable code, Rails streamlines the development process, enabling developers to swiftly build and iterate on API features. For instance, scaffolding can create a full set of endpoints for a resource in a matter of minutes, rather than hours.
- Robust Ecosystem: Rails boasts a vast ecosystem of gems (libraries) that offer ready-made solutions for common API development tasks, such as authentication, authorization, and serialization. The RubyGems repository contains over 180,000 gems, many of which are specifically designed for API development tasks. These gems significantly reduce development time and effort by providing pre-built functionalities.
- Stability and Reliability: Rails has a long history of use in production environments and is renowned for its stability and reliability. This makes it a solid choice for building APIs that demand high availability and scalability. Rails has been actively developed and used since 2003, with over 6,000 contributors contributing to its stability and feature set.
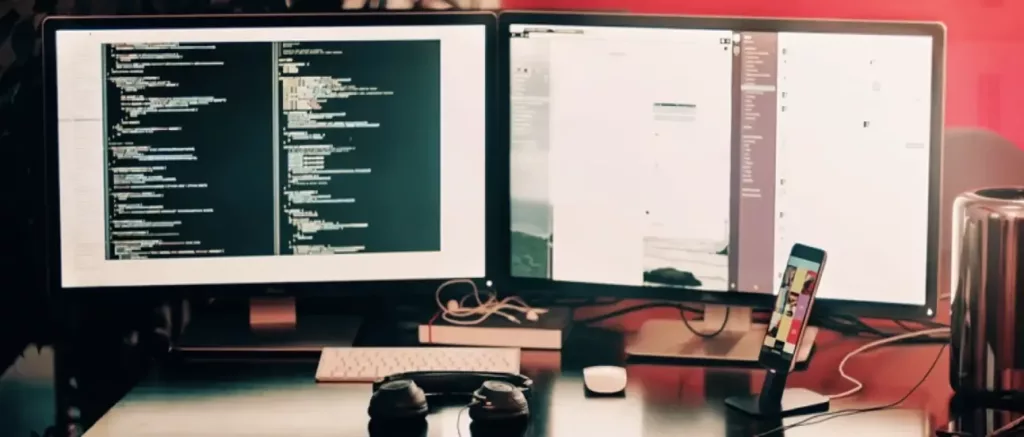
Setting Up a Rails API Application
To create a new Rails application specifically tailored for API purposes, use the following command:
Bash
rails new my_api --api --database=postgresql
This command generates a new Rails application with configurations optimized for API development:
- API Mode: The
--api flag
configures the application to exclude middleware and modules that are primarily used for browser-based applications. This results in a leaner and more efficient API server by removing unnecessary components. - PostgreSQL Database: The
--database=postgresql
flag sets up the application to use PostgreSQL as the database. PostgreSQL is a powerful and reliable open-source relational database that is well-suited for production use.
Creating API Endpoints
Rails offers a robust routing system for defining API endpoints. Here’s an example of how to define routes for a posts resource:
Ruby
# config/routes.rb
Rails.application.routes.draw do
namespace :api do
namespace :v1 do
resources :posts
end
end
end
This code defines the following RESTful API endpoints:
HTTP Verb | URL | Action | Description |
GET | /api/v1/posts | index | List all posts |
GET | /api/v1/posts/1 | show | Show a specific post |
POST | /api/v1/posts | create | Create a new post |
PUT | /api/v1/posts/1 | update | Update a specific post |
DELETE | /api/v1/posts/1 | destroy | Delete a specific post |
Implementing Controllers
Controllers are responsible for handling the logic of processing API requests and returning appropriate responses. Here’s an example of a PostsController:
Ruby
# app/controllers/api/v1/posts_controller.rb
class Api::V1::PostsController < ApplicationController
def index
posts = Post.all
render json: posts
end
def show
post = Post.find(params[:id])
render json: post
end
# ... other actions (create, update, destroy)
end
This controller defines two actions: index for listing all posts and show for displaying a specific post. The render json: method serializes the data into JSON format, a widely used standard for API communication. It’s important to note that while Rails can handle JSON parameters, it’s not automatic for all requests. To enable JSON parameter parsing, you need to include the ActionDispatch::ParamsParser middleware.
Integrating React with the Rails API
There are several approaches to integrating React with a Rails API, each with its own trade-offs:
- Two Separate Applications: This approach involves building two independent applications: one for the React frontend and one for the Rails API. The two applications communicate via API requests. This approach provides a clear separation of concerns and allows for independent development and deployment. However, it also adds complexity in terms of managing two separate codebases and ensuring proper communication between them.
- Rails App with React Library: This approach involves building a Rails application and integrating the React library into the frontend. This approach can be simpler for smaller applications and allows for more integrated development. However, it may lead to a tighter coupling between the frontend and backend, potentially making it harder to scale or maintain in the long run.
- React on Rails Gem: This gem offers a pre-built integration of React and Rails. It allows developers to pass data from Rails to React components as props and supports server-side rendering. This can simplify development and improve performance, but it also introduces a dependency on the gem and may require some adjustments to your development workflow.
Finding Example Projects
To gain a better understanding of how Rails APIs are used with React frontends, exploring real-world examples can be invaluable. GitHub hosts a variety of open-source projects that demonstrate this integration. Some notable examples include:
- PandaNote: An Evernote clone with a Rails backend and a React frontend.
- Greenhouse: A full-stack clone of Indiegogo built with Ruby on Rails and React.js.
- SocialBusinessStarter: A KickStarter clone built with Rails and React/Redux.
- Mr.Hood: A Robinhood-inspired stock trading application using Rails, PostgreSQL, React.js, and Redux.
These projects provide practical examples of how to structure your application, handle data flow, and implement features like authentication and authorization.
Authentication and Authorization
Securing your Rails API is paramount to protect sensitive data and ensure that only authorized users can access resources. Rails provides several mechanisms and tools for implementing authentication and authorization:
- Concerns: Rails concerns are a powerful way to encapsulate and reuse code related to specific functionalities, such as authentication and authorization. By using concerns, you can modularize your code and enforce security policies consistently across your application.
- Token-Based Authentication: In this approach, users authenticate with the API using tokens, typically JSON Web Tokens (JWTs). JWTs are digitally signed tokens that contain information about the user and their permissions. This approach is well-suited for stateless API applications.
- Devise: Devise is a widely used Rails gem that provides a comprehensive authentication solution. It includes features like user registration, password recovery, and session management, making it easy to add user authentication to your Rails API.
- Pundit: Pundit is another valuable Rails gem that simplifies the implementation of authorization policies. It allows you to define granular permissions for different user roles and resources, ensuring that users can only access the data and perform the actions they are authorized for.
Serialization Formats
When exchanging data between the Rails API and the React frontend, choosing an appropriate serialization format is essential. Here are some popular options:
- JSON API: JSON API is a specification for building APIs that emphasizes consistency and discoverability. It provides a standardized way to structure JSON responses, making it easier for clients to consume and understand the data.
- Active Model Serializers: Active Model Serializers is a Rails gem that offers a convention-based approach to serializing data from Active Record models. It simplifies the process of transforming database objects into JSON format for API responses.
- Fast JSON API: Developed by Netflix, Fast JSON API is a gem that prioritizes performance and efficiency in serializing JSON data. It leverages optimized algorithms and data structures to minimize serialization overhead, which is crucial for high-performance APIs.
Best Practices
Here are some best practices to consider when developing Ruby on Rails APIs for React frontends:
- Versioning: Implement API versioning to manage changes and ensure backward compatibility. This allows you to introduce new features or modify existing ones without breaking existing clients. Common versioning methods include using a version number in the URL (e.g., /api/v1/), a custom header, a URL parameter, or a subdomain.
- RESTful Conventions: Adhere to RESTful conventions for designing API endpoints. REST, or Representational State Transfer, is an architectural style that promotes a standardized approach to API design. By following RESTful principles, such as using nouns to represent resources, verbs to represent actions, and HTTP status codes to indicate the outcome of requests, you can create APIs that are easy to understand, use, and scale.
- Stateless Communication: Each request from the client to the server should contain all the necessary information to process the request, making the server independent of previous requests, i.e.: POST for creating resources, PUT for updating resources, and DELETE for deleting resources.
- Serialization: Choose an appropriate serialization format and optimize it for performance. Consider the size and complexity of your data, the performance requirements of your API, and the capabilities of your chosen serialization library.
- Authentication and Authorization: Implement robust authentication and authorization mechanisms to secure your API. This is crucial to protect sensitive data and ensure that only authorized users can access resources.
- Error Handling: Provide informative error messages to help clients understand and resolve issues. Clear and concise error messages can significantly improve the developer experience when integrating with your API.
- Documentation: Document your API clearly to make it easy for developers to understand and use. Good documentation should include information about available endpoints, request and response formats, authentication methods, and error handling.
Deployment
Deploying your Rails API is a crucial step in making it accessible to your React frontend. Heroku is a popular platform-as-a-service (PaaS) that provides an easy and efficient way to deploy Rails applications. Here’s a simple deployment process using Heroku:
- Install the Heroku CLI.
- Log in to your Heroku account.
- Create a new Heroku app.
- Push your code to Heroku.
<!– end list –>
Bash
heroku create
git push heroku main
Advanced Considerations
As your application grows in complexity, you may need to consider more advanced techniques to ensure optimal performance and maintainability:
- State Management in React: For simple state management within a component, use React’s useState hook. For more complex applications, consider using Redux or the Context API to manage state globally.
- Side Effects: Use the useEffect hook to handle side effects like fetching data or updating the DOM in your React components.
- Caching: Implement caching strategies in Rails to improve API performance. Rails provides various caching mechanisms, such as fragment caching, page caching, and action caching.
- Optimize Rendering: Use React hooks like useMemo and useCallback to prevent unnecessary re-renders of components, improving performance.
- Monitor Performance: Regularly monitor your application’s performance to identify and address potential bottlenecks. Tools like New Relic and Scout APM can provide valuable insights into your application’s performance.
Conclusion
Developing Ruby on Rails applications as an API for React frontends is a powerful approach to building modern web applications. Rails excels in handling backend logic and data management, while React provides a flexible and efficient way to create dynamic user interfaces. By combining these two frameworks and adhering to best practices for API development, you can create applications that are robust, scalable, and user-friendly. Remember to prioritize security, performance, and maintainability throughout the development process to ensure a successful and sustainable application.
As leading Ruby on Rails developers with a track record of successful projects, Vinova is your one-stop shop for building web applications. Visit us and let us bring your app ideas to life!