Ruby is a powerful and versatile programming language known for its concise syntax and flexibility. One area where Ruby truly shines is in its ability to manipulate arrays with elegant one-liners. These one-liners can save you time and make your code more readable. In this article, we’ll explore some of the most useful one-liner Ruby array tricks that every Ruby developer should know.
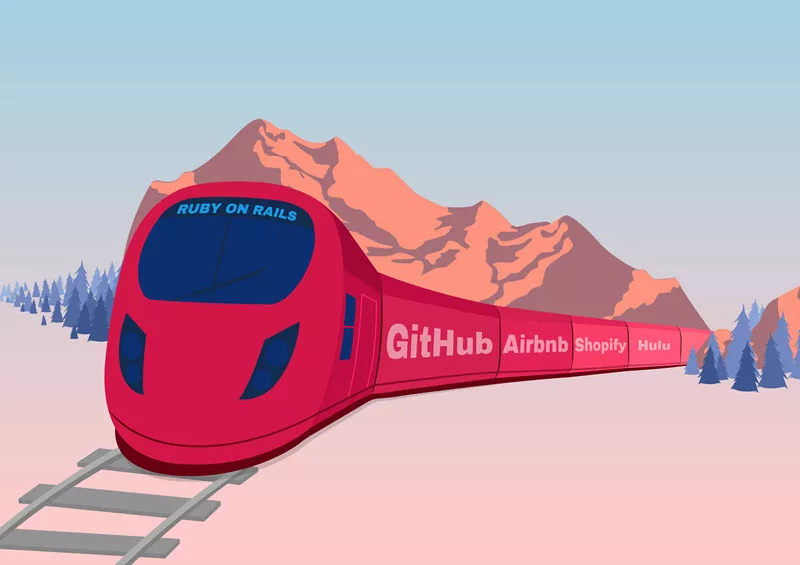
Table of Contents
Creating Arrays with One-Liners
Before we dive into manipulating arrays, let’s look at some quick ways to create them. Ruby offers several concise methods for creating arrays on the fly:
- Using
%w
: This is a handy way to create an array of strings without all the quotes and commas. For example,%w{apple banana cherry}
creates the array [“apple”, “banana”, “cherry”]. - Using
*
: You can use the splat operator to create an array with the same element repeated multiple times. For instance,*5
will produce the array [5, 5, 5, 5, 5]. - Using Ranges: Ranges provide a convenient way to generate sequences of numbers.
(1..5).to_a
creates the array [1, 2, 3, 4, 5], and you can even create arrays with specific steps like(2..10).step(2).to_a
to get [2, 4, 6, 8, 10].
Transforming Elements with map
The map method is a workhorse when it comes to array manipulation in Ruby. It allows you to iterate over an array and apply a transformation to each element, returning a new array with the transformed values. Here’s the basic syntax:
Ruby
array.map { |element| transformation }
For instance, if you want to square each number in an array, you can do it in a single line:
Ruby
numbers = [1, 2, 3, 4, 5]
squares = numbers.map { |number| number * number } #=> [1, 4, 9, 16, 25]
But the real magic of map lies in its ability to work with any kind of transformation. You can use it to convert data types, extract information, or perform complex calculations. For example, let’s say you have an array of strings and you want to get the length of each string:
Ruby
words = ["apple", "banana", "cherry"]
lengths = words.map { |word| word.length } #=> [5, 6, 6]
The splat operator (*) can be particularly useful when used in conjunction with map. For example, you can use it to pass an array of arguments to a method that expects multiple arguments:
Ruby
def greet(name, age)
Hello, Alice! You are 30 years old.
puts "Hello, #{name}! You are #{age} years old."
end
user_data = ["Alice", 30]
greet(*user_data) # Output:
Filtering with select and reject
The select and reject methods are your go-to tools for filtering arrays. select creates a new array containing only the elements that meet a certain condition, while reject does the opposite, returning an array with the elements that don’t satisfy the condition.
Here’s how you can use select to get all the even numbers from an array:
Ruby
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = numbers.select { |number| number.even? } #=> [2, 4, 6]
And here’s how you can use reject to remove all the odd numbers:
Ruby
numbers = [1, 2, 3, 4, 5, 6]
odd_numbers = numbers.reject { |number| number.even? } #=> [1, 3, 5]
Flattening with flatten and flat_map
When you’re dealing with nested arrays, flatten and flat_map can be incredibly helpful. flatten takes a multi-dimensional array and converts it into a one-dimensional array.
Ruby
nested_array =, [3, 4], [5, 6]]
flat_array = nested_array.flatten #=> [1, 2, 3, 4, 5, 6]
flat_map is similar, but it also allows you to apply a transformation to each element before flattening the array. Here’s a simple example:
Ruby
array =, [3, 4]]
array.flat_map { |subarray| subarray + [5] } #=> [1, 2, 5, 3, 4, 5]
This is particularly useful when you want to extract information from nested arrays. For example:
Ruby
nested_array =, [3, 4], [5, 6]]
transformed_array = nested_array.flat_map { |subarray| subarray.map { |number| number * 2 } } #=> [2, 4, 6, 8, 10, 12]
Finding Elements with find and detect
The find and detect methods are used to find the first element in an array that satisfies a given condition. They are essentially aliases of each other.
Ruby
numbers = [1, 2, 3, 4, 5]
first_even_number = numbers.find { |number| number.even? } #=> 2
Counting with count
The count method is a simple but effective way to count the number of elements in an array that meet a certain condition.
Ruby
numbers = [1, 2, 3, 4, 5]
even_count = numbers.count { |number| number.even? } #=> 2
Reducing with reduce and inject
The reduce and inject methods are powerful tools for performing calculations on arrays. They allow you to iterate over an array and accumulate a value based on each element.
For example, you can use reduce to sum all the numbers in an array:
Ruby
numbers = [1, 2, 3, 4, 5]
sum = numbers.reduce { |sum, number| sum + number } #=> 15
Working with Indices with each_with_index
The each_with_index method is a handy way to iterate over an array and access both the element and its index at the same time.
Ruby
fruits = ["apple", "banana", "cherry"]
fruits.each_with_index { |fruit, index| puts "#{index}: #{fruit}" }
This will output:
0: apple
1: banana
2: cherry
Chaining Methods
One of the most powerful features of Ruby array methods is the ability to chain them together. This allows you to perform multiple operations on an array in a single line of code.
For example, you can chain map, select, and count to find the number of even squares in an array:
Ruby
numbers = [1, 2, 3, 4, 5]
even_square_count = numbers.map { |number| number * number }.select { |number| number.even? }.count #=> 2
While chaining is convenient for readability and conciseness, it’s important to be mindful of potential performance implications. In some cases, especially with complex or performance-critical operations, using a traditional loop might be more efficient than excessive chaining3.
Method References
Ruby provides a shorthand syntax for calling methods on array elements using method references. This can make your code more concise and readable.
For example, instead of writing:
Ruby
numbers = [1, 2, 3, 4]
strings = numbers.map { |number| number.to_s }
You can simply write:
Ruby
numbers = [1, 2, 3, 4]
strings = numbers.map(&:to_s)
This shorthand syntax is actually a clever use of Ruby’s Symbol#to_proc
m33ethod. &:to_s
is equivalent to &:to_s.to_proc
. The to_proc
method on a symbol converts it into a proc that, when called, invokes the method with the same name on the given object.
Numbered Arguments
Since Ruby 2.7, you can use numbered arguments within blocks to further simplify your one-liners. This is especially useful when working with methods like each_with_index
.
For example, instead of writing:
Ruby
fruits = ["apple", "banana", "cherry"]
fruits.each_with_index { |fruit, index| puts "#{index}: #{fruit}" }
You can write:
Ruby
fruits = ["apple", "banana", "cherry"]
fruits.each_with_index { puts "#{_2}: #{_1}" }
Grouping with group_by
The group_by method allows you to group elements in an array based on a specific criterion. This can be useful for organizing data and performing calculations on groups of elements.
For example, you can group an array of words by their first letter:
Ruby
words = ["apple", "banana", "avocado", "cherry"]
grouped_words = words.group_by { |word| word[0] } #=> {"a"=>["apple", "avocado"], "b"=>["banana"], "c"=>["cherry"]}
Extracting Elements with values_at
The values_at method lets you pick out elements from an array using their indices. For example, [1, 2, 3, 4].values_at(0, 2) would return.
Destructive and Non-Destructive Methods
It’s important to be aware that some Ruby array methods are “destructive,” meaning they modify the original array, while others are “non-destructive” and return a new array with the changes.
For example, sort returns a new sorted array, while sort! sorts the array in place. Similarly, uniq returns a new array with duplicates removed, while uniq! removes duplicates from the original array.
When choosing between destructive and non-destructive methods, consider whether you need to preserve the original array or if modifying it directly is acceptable.
Conclusion
These one-liner Ruby array tricks demonstrate the power and expressiveness of Ruby. By mastering these techniques, you can write cleaner, more readable, and more efficient Ruby code. Concise one-liners can improve code readability and reduce the need for verbose loops or temporary variables. However, it’s also crucial to understand the potential performance implications of excessive method chaining and to choose between destructive and non-destructive methods appropriately.
I encourage you to explore the Ruby section on Vinova’s blog further to discover even more powerful one-liners and to practice using these techniques in your own code.