Is your Ruby on Rails API struggling under load? Often, the bottleneck lies in serialization. Meet jsonapi-serializer (formerly Fast JSON API), a gem laser-focused on optimizing this exact process. Born from performance needs at Netflix, it tackles slow JSON generation head-on. The difference?
Benchmarks show it can be significantly faster than libraries like Active Model Serializer (AMS), with reports of speed gains exceeding 25x in certain scenarios—think serializing 250 records in ~3ms versus AMS’s ~138ms. Adhering strictly to the JSON:API spec, it offers both speed and structure. Let’s explore how it delivers these results.
Table of Contents
1. What Is Fast JSON API?
Fast JSON API is a high-performance Ruby gem designed for efficient JSON:API serialization in Rails applications, offering a speed-focused alternative to Active Model Serializer (AMS). It improves API response times, particularly under heavy data loads, by defining serializer classes that structure JSON output separately from controllers, enhancing code organization.
Strictly adhering to the JSON:API specification, Fast JSON API ensures standardized response formats, benefiting frontend applications with predictable data structures. However, its nested format may feel verbose for simpler APIs.
Originally developed at Netflix, the gem addresses serialization challenges in data-intensive applications. Benchmarks show Fast JSON API over 25x faster than AMS in some cases, rendering 250 records in ~3ms vs. AMS’s 138ms. Performance improvements range from 4x to 25x, depending on the task and dataset size.
Its speed and efficiency make Fast JSON API a strong choice for optimizing Rails API performance.
Considering these performance figures and the standardized output, when does integrating Fast JSON API become the right technical decision for your Rails project?
2. Core Functionalities of Fast JSON API
Fast JSON API provides fine-grained control over your JSON:API output.
Use the attributes method in your serializer to select which model attributes appear in the response. While it defaults to matching model property names, you can use blocks for computed values, data transformation, or renaming attributes, effectively decoupling your API structure from your data model. Conditional attributes allow context-specific output.
Similarly, map ActiveRecord associations (belongs_to, has_many, has_one) directly within the serializer. By default, relationships only include the id and type of related objects, encouraging smaller initial API payloads. To embed full related resource details (sideloading), leverage the compound documents feature. The gem also supports customizing relationship output, including conditional inclusion.
Key transforms modify attribute and relationship names in the final JSON. This is commonly used to shift from Ruby’s snake_case to JavaScript’s preferred camelCase, aligning with Active Model Serializer’s transformation options.
Beyond attributes and relationships, Fast JSON API includes several helpful tools:
- Serializer Generation: Focusing specifically on the `rails generate serializer` command and how it bootstraps the process.
- Performance Optimization Features: Detailing the caching mechanism (how it works, typical benefits) and the Skylight instrumentation.
- JSON:API Specification Adherence Features: Expanding on Links (hypermedia), Meta-information, and Sparse Fieldsets, explaining why these are important parts of the JSON:API standard and how Fast JSON API supports them.
- Handling Collections: Discussing the specific options Fast JSON API provides for serializing arrays or lists of objects.
- Deserialization (via the `jsonapi-serializer` fork): Explaining the purpose and benefit of parsing incoming JSON:API data, which wasn’t part of the original gem but is crucial for full API interactions.
With these distinct mechanisms for attributes, relationships, naming, and performance optimization, how do you determine the most effective combination for your specific API requirements?
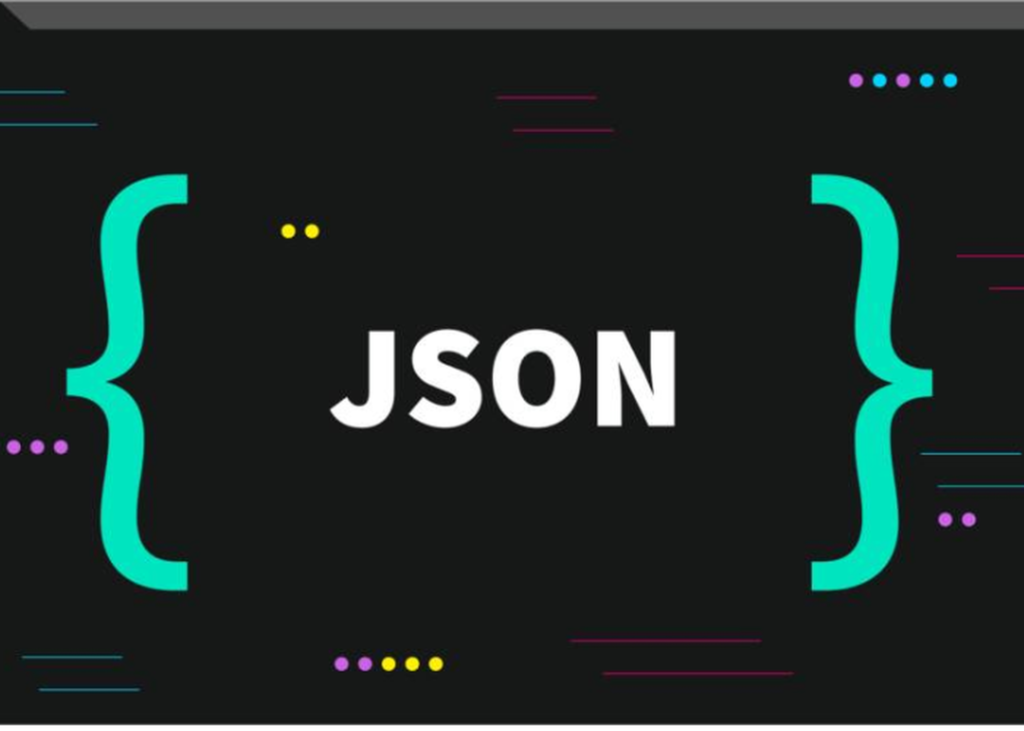
3. Performance Analysis
The decision often hinges on performance when comparing Fast JSON API to alternatives like Active Model Serializer (AMS). Let’s examine the numbers.
Serialization Speed
Direct performance tests, often included within the gem’s own repository (originally Fast JSON API, now the jsonapi-serializer fork), consistently highlight significant speed advantages.
- Benchmark Results: Comparisons regularly show Fast JSON API completing serialization tasks much faster than AMS. A frequently cited metric is a speed improvement of 25 times or more. These tests typically cover scenarios processing varying numbers of records (e.g., 1, 25, 250, 1000) to assess scalability.
- Specific Example: One benchmark measuring the creation of a Ruby hash for 250 records reported AMS taking 138.71 milliseconds, while Fast JSON API completed the task in just 3.01 milliseconds. That translates to an approximate 46x speed increase for that specific operation. Another independent test showed serialization time dropping from 38.8ms (AMS) to 5.9ms (Fast JSON API) for a set of articles.
- Underlying Factors: These gains are often attributed to Fast JSON API’s focused design, specifically optimizing for the JSON:API standard. This specialization, stemming from Netflix’s original need to address performance issues with AMS for consistent JSON:API output and homogenous object lists, likely allows for more efficient internal processing compared to AMS’s more general-purpose approach. Benchmarks may measure only hash generation or extend to include final JSON string conversion.
Resource Consumption
Beyond raw speed, CPU and memory usage matter for API efficiency.
- Memory Efficiency: While comprehensive benchmarks comparing memory footprints directly are less common than speed tests, anecdotal reports suggest Fast JSON API can be more memory-efficient. One user specifically noted a substantial decrease in average memory consumption after switching from AMS.
- Context is Key: It is important to note that actual resource usage depends heavily on the specific application, data structures, and traffic patterns. Detailed profiling within your own environment is necessary for definitive resource consumption figures.
The potential for drastically faster serialization times, sometimes exceeding 25x improvements, coupled with indications of better memory efficiency, makes a strong case for Fast JSON API in performance-sensitive applications.
With these quantifiable speed gains and potential resource savings in mind, how might these performance characteristics influence the architecture and scalability of your next Rails API project?
This table shows that Fast JSON API can significantly improve the performance of Ruby on Rails applications by greatly reducing JSON serialization time:
Metric | Active Model Serializer (Example) | Fast JSON API (Example) | Improvement Factor | Source(s) | Notes |
Serialization Time (250 records) | 138.71 ms | 3.01 ms | ~46x | 6 | Ruby Hash Generation |
Serialization Time (Collection of Articles) | 38.8 ms | 5.9 ms | ~6.5x | 15 | JSON String Generation |
Memory Usage (Anecdotal) | Higher | Lower | Significant | 26 | Context-dependent, specific numbers vary |
4. Implementing Fast JSON API in Ruby on Rails
Integrating the jsonapi-serializer gem (the successor to fast_jsonapi) into your Rails application follows a clear path, enabling you to leverage its performance benefits—recall the benchmarks showing potential speed improvements of over 25x compared to alternatives like AMS in certain scenarios.
1. Installation
First, ensure the gem is part of your project’s dependencies. Add this line to your Gemfile:
gem ‘jsonapi-serializer’
Then, run bundle install from your terminal within the application directory to install the gem.
2. Generating Serializer Classes
The gem includes a Rails generator to quickly create serializer files. Use the following command structure:
rails g serializer <ModelName> <attribute1> <attribute2> …
For example, to set up a serializer for a User model including specific attributes:
rails g serializer User id email name registered_at
This command creates a new file, typically app/serializers/user_serializer.rb, pre-populated with the necessary boilerplate including JSONAPI::Serializer.
3. Defining Serialization Logic
Open the generated serializer file (e.g., app/serializers/user_serializer.rb). Here, you define precisely what data gets included:
- Use the attributes method followed by symbol names for each model attribute you want in the output (e.g., attributes :email, :name, :registered_at). The :id is usually handled automatically.
- Define relationships using belongs_to, has_many, or has_one (e.g., belongs_to :account, has_many :posts).
4. Controller Integration
Finally, use the serializer within your controller actions to format the JSON response:
Single Object: Instantiate the serializer with the object and call .serializable_hash.
# Example: users_controller.rb
def show
@user = User.find(params[:id])
render json: UserSerializer.new(@user).serializable_hash
end
- IGNORE_WHEN_COPYING_START
content_copy download
Use code with caution.Ruby
IGNORE_WHEN_COPYING_END
Collection of Objects: The pattern is identical for collections.
# Example: users_controller.rb
def index
@users = User.all
render json: UserSerializer.new(@users).serializable_hash
end
- IGNORE_WHEN_COPYING_START
content_copy download
Use code with caution.Ruby
IGNORE_WHEN_COPYING_END
Including Related Data (Compound Documents): To embed related resources (sideloading), use the include: option during instantiation. Pass an array of relationship names.
# Example: users_controller.rb
def show
@user = User.find(params[:id])
options = { include: [:posts, :account] } # Include related posts and account
render json: UserSerializer.new(@user, options).serializable_hash
end
- IGNORE_WHEN_COPYING_START
content_copy download
Use code with caution.Ruby
IGNORE_WHEN_COPYING_END
Calling .serializable_hash explicitly constructs the JSON:API-compliant hash structure before Rails converts it to the final JSON string.
With the serializer installed, generated, defined, and integrated, your API is now equipped to serialize data according to the JSON:API standard efficiently. But what advanced configurations or potential edge cases should you consider as your API complexity grows?
5. Handling Compound Documents (Sideloading)
A core optimization technique within the JSON:API specification is the use of compound documents, commonly called “sideloading.” Its purpose is straightforward: minimize the number of HTTP requests a client needs to make to fetch related data. Instead of requesting a primary resource and then making follow-up requests for its associations, the server can embed these related resources directly into the initial response. This efficiency builds upon the inherent speed advantages of Fast JSON API (recall those benchmarks showing potential serialization speed-ups exceeding 25x).
Implementing Sideloading in Fast JSON API
The mechanism is primarily handled via the include option passed when initializing the serializer in your controller:
Specify Relationships: Provide an array of relationship names (as defined in your serializer) to the include: option.
# Example: posts_controller.rb
def show
@post = Post.find(params[:id])
# Include the post’s author and comments
options = { include: [:author, :comments] }
render json: PostSerializer.new(@post, options).serializable_hash
end
Nested Includes: You can also include relationships nested deeper within the hierarchy using dot notation in strings.
# Include comments AND the author of each comment
options = { include: [‘comments.author’] }
render json: PostSerializer.new(@post, options).serializable_hash
-
IGNORE_WHEN_COPYING_START
content_copy download
Use code with caution.Ruby
IGNORE_WHEN_COPYING_END - Prerequisite: Define Related Serializers: For Fast JSON API to serialize included resources correctly, you must have corresponding serializer classes defined for each included relationship type (e.g., AuthorSerializer, CommentSerializer). The gem automatically detects and uses these.
Understanding the Response Structure
When using include, the JSON response structure changes:
- data: Contains the primary resource(s) as usual.
- included: A new top-level array appears. This array holds the fully serialized related resources requested via the include option. Each item in included will have its own id, type, attributes, and potentially its own relationships, formatted by its respective serializer.
- Relationships Links: Within the primary resource’s relationships block in the data section, the associated resources will now link (via id and type) to their corresponding entries in the included array, rather than embedding the full data directly there.
This standardized approach ensures predictability for API consumers and significantly reduces network chatter by bundling related data efficiently.
While include handles what related data to fetch, what if you need finer control over how that included data is presented or want to conditionally include relationships based on context?
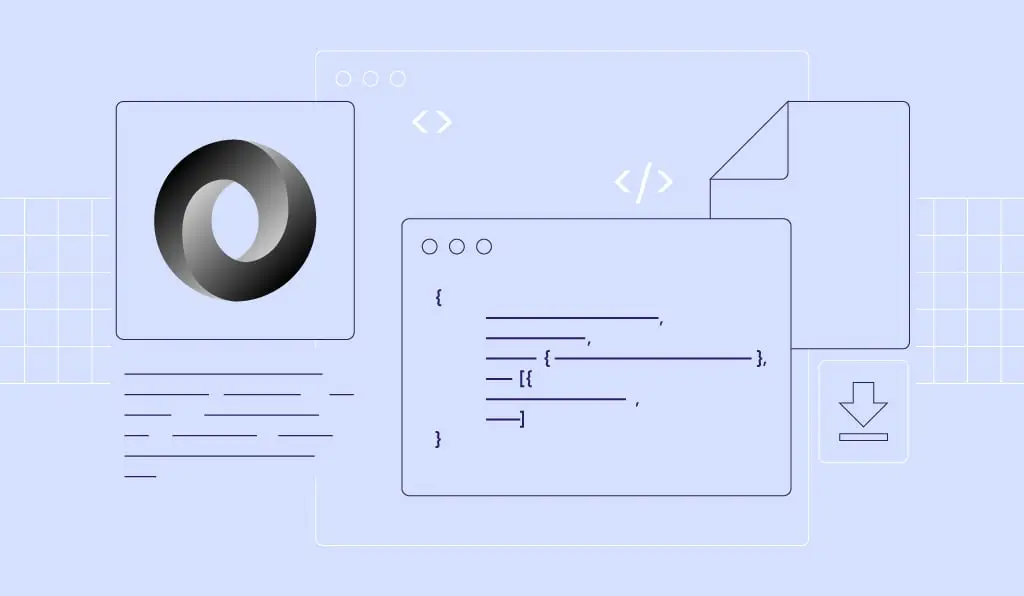
6. Best Practices and Optimization Techniques
Leveraging Fast JSON API (or its successor, jsonapi-serializer) delivers significant serialization speed gains—recall benchmarks indicating improvements exceeding 25x in some cases. However, maximizing overall API performance and maintainability requires adopting specific practices.
1. Be Selective with Attributes
Only include attributes genuinely needed by the API consumer. Every extra attribute increases JSON payload size, consuming bandwidth and adding client-side processing overhead. Crucially, avoid inadvertently exposing sensitive internal model data through the API. Review your attributes declarations regularly.
2. Mind Your Includes (Sideloading)
Compound documents (include: option) are powerful for reducing HTTP requests, but use them judiciously. Including too many relationships, especially nested ones, can lead to “over-fetching”—sending large amounts of data the client doesn’t actually need. Analyze each endpoint’s requirements: is sideloading more efficient than letting the client make specific follow-up requests?
3. Leverage Caching
Fast JSON API’s built-in caching mechanism can dramatically reduce serialization load for frequently accessed, infrequently changed data. By default, it often uses the model’s cache_key for invalidation. Implement proper cache expiration strategies. For more demanding scenarios, consider integrating dedicated cache stores like Redis or Memcached to further offload work from your application servers. Avoiding serialization altogether, even fast serialization, is a significant win.
4. Optimize Database Queries
Fast serialization cannot compensate for slow database interactions. This is often the real bottleneck.
- Prevent N+1 Queries: Use ActiveRecord’s includes (eager loading) in your controller before passing data to the serializer, especially when using the serializer’s include option. Failure to do so can result in numerous database queries for a single API request.
- Select Specific Columns: Use select in your queries to fetch only the columns required by the serializer and your application logic, reducing data transfer from the database and memory usage.
5. Enhance Serializer Maintainability
- Use Helper Methods: Extract common formatting logic or complex data transformations within your serializer into private helper methods for cleaner, more reusable code.
- Apply Conditional Logic: Employ conditional attributes (attribute … if: -> { … }) and relationships to tailor responses based on request parameters or user permissions, avoiding unnecessary data exposure or computation.
6. Know When Other Tools Might Fit Better
Fast JSON API excels at standardized, performant JSON:API serialization. However:
- For APIs where clients need fine-grained control over requested data fields, GraphQL might be a more suitable paradigm.
- For very simple APIs not needing the structure or features of JSON:API, basic Rails render json: object.to_json or the Jbuilder gem could suffice with less overhead.
Remember, optimizing an API involves a holistic view—considering database performance, caching strategies, network latency, and efficient serialization together.
Having implemented these foundational best practices, what specific advanced features or edge-case scenarios might you encounter as you scale your Fast JSON API-powered application?
7. Case Studies and Open-Source Examples
The performance improvements attributed to Fast JSON API aren’t just theoretical; real-world implementations provide tangible evidence.
- Netflix: The Origin Story: Netflix, the original developer, stands as the most prominent case. Facing performance bottlenecks with Active Model Serializer (AMS) in their high-traffic Rails APIs, they engineered Fast JSON API specifically to address these issues. Their internal adoption and reliance on the gem underscore its capability to handle demanding, large-scale production environments. This origin provides direct validation of its potential performance gains—gains quantified in benchmarks showing speed increases often exceeding 25 times compared to AMS in specific serialization tasks.
- Soundstripe’s Migration: The engineering team at Soundstripe publicly documented their migration from AMS to Fast JSON API. Their report detailed noticeable performance enhancements post-migration, offering insights into the practical steps and benefits achieved in a production setting outside the originating company.
- Community Uptake (jsonapi-serializer): While the provided information doesn’t detail numerous large-scale open-source projects using the original fast_jsonapi gem by name, the existence and active maintenance of its fork, jsonapi-serializer, signals continued relevance and adoption within the developer community. Examining dependencies on platforms like GitHub can reveal projects utilizing this performant serialization approach.
- Illustrative Tutorials: Resources like the tutorial by BoTree Technologies offer practical demonstrations comparing AMS and Fast JSON API within sample Rails applications. These examples clearly illustrate the significant speed differences in controlled settings, reinforcing the benchmark data.
The foundational case—Netflix’s need for speed leading to Fast JSON API’s creation—combined with reports like Soundstripe’s migration, provides compelling indicators of its effectiveness.
Seeing these examples and the documented performance leaps, what specific metrics or thresholds in your own API’s performance would trigger consideration of such a migration?
8. Conclusion
Fast JSON API, actively developed as jsonapi-serializer, offers a focused solution for Ruby on Rails API serialization. Its standout feature remains its performance. Benchmarks consistently demonstrate significantly faster serialization compared to alternatives like Active Model Serializer (AMS)—often cited as being over 25 times faster in specific test cases—making it a strong candidate for APIs where millisecond response times matter. Coupled with its strict adherence to the JSON:API specification, it provides a predictable, standardized output format valuable for interoperability, particularly with frontend frameworks expecting this structure.
Decision Factors:
Choose Fast JSON API when: | Consider Alternatives when: |
API response speed is a primary operational concern, especially under high load or with large datasets. | The inherent structure and potential verbosity of JSON:API are unsuitable for your use case (e.g., simple internal APIs). |
Strict adherence to the JSON:API standard is required or beneficial for your ecosystem. | Maximum flexibility in JSON structure is needed (consider Jbuilder or basic to_json). |
Your team finds its declarative syntax (similar to AMS) efficient for development. | Client-driven data fetching shapes (like varying fieldsets per request) are paramount (GraphQL might be a better fit). |
Staying updated with the jsonapi-serializer releases ensures access to the latest optimizations. Always benchmark within your application’s context to validate performance gains, as real-world results depend on your specific data and query patterns.
Understanding these trade-offs is key to building efficient, maintainable APIs. Explore more deep dives into Rails performance and API design strategies on our tech blog.